Starting with C++ How C++ evolved from C? The history of C++ begins with C. C++ is built upon the foundation of C. Thus, C++ is a superset of C. C++ expanded and enhanced the C language to support objectoriented programming. C++ also added several other improvements to the C language, including an extended set […]
Programming In C++ tutorials
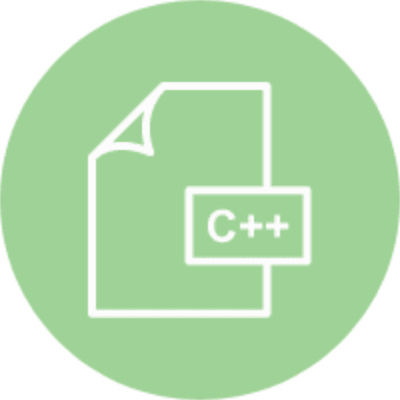
Before Moving Ahead with the Programming In C++ tutorials, Lets have a look at what we will learn in these Topics.
By the end of this Subject, we will be able to learn:
In the procedure oriented approach, the problem is viewed as the sequence of things to be done such as reading, calculating and printing such as C, Pascal, fortran etc. The primary focus is on functions. A typical structure for procedural programming is shown in fig below. The technique of hierarchical decomposition has been used to […]
Here is an example of a complete C++ program: // The C++ compiler ignores comments which start with // double slashes like this, up to the end of the line. /* Comments can also be written starting with a slash followed by a star, and ending with a star followed by a slash. As you can see, […]
Built-in-type 1) Integral type : – The data types in this type are int and char. The modifiers signed, unsigned, long & short may be applied to character & integer basic data type. The size of int is 2 bytes and char is 1 byte. 2) void – void is used : i) To specify the return […]
Declaration of variables. C requires all the variables to be defined at the beginning of a scope. But c++ allows the declaration of variable anywhere in the scope. That means a variable can be declared right at the place of its first use. It makes the program easier to understand. In order to use a […]
Assignment (=): The assignment operator assigns a value to a variable.a=5; This statement assigns the integer value 5 to the variable a. The part at the left of the assignment operator (=) is known as the lvalue (left value) and the right one as the rvalue (right value). The lvalue has to be a variable […]
IF – ELSE STATEMENT The complete form of the if statement is if(expression) statement else statement where the targets of the if and else are single statements. The else clause is optional. The targets of both the if and else can also be blocks of statements. The general form of the if using blocks of statements is […]
Advantages of OOP languages are: (i) OOP introduces the concept of data hiding & data encapsulation, because of which user is exposed to minimal data, thus creating safer programs. (ii) OOP introduces the concept of data abstraction because of which the user can only see the required features and does not need to go background […]
Class is a collective representation of a set of member variable and member function under one name. Structure is a collection of member variables and function of various data types. Difference between Class and Structure Class • By default the member of the classes are private. Structure • By default the members of Structures are public.
It is a non-member function that can access the private members of the class to which it is declared as friend. According to OOP concept of data hiding or data encapsulation, no outside function can access the private data in a class. Since friend function is a clear violation of this concept. It is rarely […]
What is class? Class is a collection of similar types of objects, where each class having similar properties and types. Ex: Bird, Animal Need of class and object Class supports data encapsulation and data abstraction which leads C++ a more secure programming language. Apart from these features, the class also has private and public section, […]
Member functions: All the the declared functions and varibles are part of the class and hence members of the corresponding class. Those are declared and defined outside the class are known as not the members of the class. So all the functions declared inside the class are known as member functions. Ex: #include <iostream> Using […]
Static members Class members which are declared as static are known as Static members. Always Static variable shares same memory location. Syntax: Static int a; Static void show(); Static Variables The data members which are declared static are known as static variables. #include <iostream> using namespace std; class num { static int c; public: void […]
One of the best feature that OOP provides is inheritance, where a new class can be constructed from an existing class. The class which is newly constructed is known as derived class and the class from which new class is constructed is known as Base class. Derived classes We can derive as many new classes […]
Polymorphism Poly means many and morphism means form and this is a greek word. In OOP function overloading and operating are example of polymorphism. Polymorphism means one name multiple form. Note: Virtual function is also another example of polymorphism which we will teach you later. Polymorphism is of two types i.e. static polymorphism or early […]
A constructor (having the same name as that of the class) is a member function which is automatically used to initialize the objects of the class type with legal initial values. Destructors are the functions that are complimentary to constructors. These are used to deinitialize objects when they are destroyed. A destructor is called when […]
There are different type of constructors in C++ 1 Overloaded Constructors Besides performing the role of member data initialization, constructors are no different from other functions. This included overloading also. In fact, it is very common to find overloaded constructors. For example, consider the following program with overloaded constructors for the figure class //Illustration of […]
The syntax for declaring a destructor is : -name_of_the_class() { } So the name of the class and destructor is same but it is prefixed with a ~ (tilde). It does not take any parameter nor does it return any value. Overloading a destructor is not possible and can be explicitly invoked. In other words, […]
class to which it (the operator) is applied. The operator function helps us in doing so. The Syntax of declaration of an Operator function is as follows: Operator Operator_name For example, suppose that we want to declare an Operator function for ‘=’. We can do it as follows: operator = A Binary Operator can be defined either […]
C++ supports two complete I/O systems: the one inherited from C and the object oriented I/O system defined by C++ (hereafter called simply the C++ I/O system). Like C-based I/O, C++’s I/O system is fully integrated. The different aspects of C++’s I/O system, such as console I/O and disk I/O, are actually just different perspectives on the same mechanism.Every […]
The classes istream and ostream define two member functions get(),put() respectively to handle the single character input/output operations. There are two types of get() functions.Both get(char *) and get(void) prototype can be used to fetch a character including the blank space,tab and newline character. The get(char *) version assigns the input character to its argument and the […]
A line of text can be read or display effectively using the line oriented input/output functions getline() and write(). The getline() function reads a whole line of text that ends with a newline character.This function can be invoked by using the object cin as follows: cin.getline(line,size); This function call invokes the function getline() which reads […]
When a large amount of data is to be handled in such situations floppy disk or hard disk are needed to store the data .The data is stored in these devices using the concept of files. A file is a collection of related data stored in a particular area on a disk. Programs can be designed to perform […]
While using constructor for opening files,filename is used to initialize the file stream object. This involves the following steps Create a file stream object to manage the stream using the appropriate class i.e the class ofstream is used to create the output stream and the class ifstream to create the input stream. Initialize the file […]
The function open() can be used to open multiple files that uses the same stream object. For example to process a set of files sequentially,in such case a single stream object can be created and can be used to open each file in turn. This can be done as follows; File-stream-class stream-object; stream-object.open (“filename”); The following example […]