OOP Using C++ – C++ Programming Templates ,Exceptions and STL based Questions and Answers
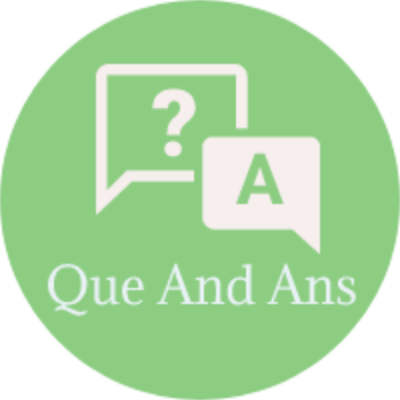
Certainly! Here’s a list of 100 C++ programming questions that cover templates, exceptions, and the Standard Template Library (STL).
Remember, these questions are meant to test your knowledge and understanding of templates, exceptions, and the STL in C++. Feel free to use them for practice or as a reference while studying these topics.
Templates:
1. Explain what templates are in C++ and how they are used.
In C++, templates are a powerful feature that allows for generic programming. Templates enable the creation of functions and classes that can work with different data types without sacrificing type safety. They provide a way to write reusable code that can be customized for various data types at compile-time.
Templates are defined using the `template` keyword, followed by a template parameter list. The template parameters can be either type parameters or non-type parameters, allowing for flexibility in defining generic code.
Templates can be used to create two main entities: function templates and class templates.
1. Function Templates:
Function templates are used to define generic functions that can operate on different types. They allow you to define a single function template that can be instantiated with different types when used. The compiler generates the appropriate function code for each type used with the template.
Here’s an example of a function template that calculates the square of a number:
template <typename T> T square(T num) { return num * num; }
In the above example, the `square` function template is defined with a type parameter `T`. It can be used with any valid type. When the function is called, the compiler generates the appropriate code for the specific type used.
2. Class Templates:
Class templates allow you to define generic classes that can be used with different data types. Similar to function templates, class templates provide a blueprint for generating classes for different types at compile-time. The compiler generates a specific class for each type used with the template.
Here’s an example of a class template that represents a generic Stack:
template <typename T> class Stack { private: std::vector<T> data; public: void push(T item) { data.push_back(item); } T pop() { T item = data.back(); data.pop_back(); return item; } };
In the above example, the `Stack` class template is defined with a type parameter `T`. It is used to create a stack that can store elements of any valid type. The specific implementation of the class is generated by the compiler for each type used.
To use a function or class template, you need to provide the template arguments when calling or instantiating the template. The compiler then generates the appropriate code based on the provided type or types.
int main() { // Using the function template int squaredInt = square(5); // square<int>(5) double squaredDouble = square(3.14); // square<double>(3.14) // Using the class template Stack<int> intStack; intStack.push(10); intStack.push(20); int poppedItem = intStack.pop(); return 0; }
In the above example, the function template `square` is used with both `int` and `double` types. The compiler generates the appropriate function code for each type used.
The class template `Stack` is instantiated with the `int` type, creating an `int` stack. The `push` and `pop` functions of the `intStack` object operate on `int` values.
Templates are a powerful tool in C++ that enable code reuse and provide flexibility in working with different data types. They allow for generic programming, where algorithms and data structures can be designed independently of specific types, enhancing code modularity and maintainability.
2. What is the difference between function templates and class templates?
The main difference between function templates and class templates in C++ lies in their purpose and usage:
1. Function Templates:
Function templates are used to define generic functions that can operate on multiple data types. They allow you to define a single function template that can be instantiated with different types when used. The compiler generates the appropriate function code for each type used with the template.
- Function templates are typically used when you want to perform the same logic or operation on different types of data.
- They are defined using the `template` keyword followed by a template parameter list, which includes type parameters or non-type parameters.
- When a function template is called, the compiler deduces the types of the template arguments and generates the corresponding function code.
- Example: A function template to calculate the square of a number can be used with `int`, `double`, or any other numeric type.
2. Class Templates:
Class templates allow you to define generic classes that can be instantiated with different data types. They provide a blueprint for generating classes for different types at compile-time. The compiler generates a specific class for each type used with the template.
- Class templates are used when you want to define a generic class that can work with various data types.
- They are defined using the `template` keyword followed by a template parameter list, which includes type parameters or non-type parameters.
- When a class template is instantiated, the compiler generates a specific class based on the provided type or types.
- Example: A class template for a container, such as a stack or a linked list, can be used to create container objects for different data types, such as `int`, `double`, or custom types.
In summary, function templates are used to define generic functions that can operate on different types, while class templates are used to define generic classes that can work with various data types. Function templates allow you to define a single function that can be used with different types, whereas class templates allow you to define a single class that can be instantiated with different types to create specific instances of the class.
3. Write a template function to find the maximum of two numbers.
Certainly! Here’s an example of a template function to find the maximum of two numbers:
template <typename T> T findMax(T a, T b) { return (a > b) ? a : b; }
In the above example, the `findMax` function is defined as a template function using the `template` keyword and the type parameter `T`. The function takes two parameters `a` and `b` of type `T`, which can be any comparable data type, such as `int`, `double`, or `float`.
The function body compares the values of `a` and `b` using the `>` operator. If `a` is greater than `b`, it returns `a`; otherwise, it returns `b`. The ternary conditional operator (`?:`) is used to express this comparison in a concise manner.
You can use this template function to find the maximum of two numbers as follows:
int main() { int maxInt = findMax(10, 20); double maxDouble = findMax(3.14, 2.71); std::cout << "Maximum integer: " << maxInt << std::endl; std::cout << "Maximum double: " << maxDouble << std::endl; return 0; }
In the `main()` function, the `findMax` function is called with different arguments of the same type. The compiler deduces the type of the template argument based on the provided arguments.
The returned maximum value is then stored in variables `maxInt` and `maxDouble`. Finally, the results are printed to the console.
Output: Maximum integer: 20 Maximum double: 3.14
By using this template function, you can find the maximum of two numbers regardless of their specific type.
4. How can you specialize a template function or class for a specific type?
In C++, you can specialize a template function or class to provide a specific implementation for a particular type or set of types. Template specialization allows you to customize the behavior of a template when it is used with specific template arguments.
There are two types of template specialization: explicit specialization and partial specialization.
1. Explicit specialization:
Explicit specialization allows you to provide a specialized implementation for a specific type. It involves explicitly defining the template function or class for the desired type.
Here’s an example of explicit specialization for a template function:
template <typename T> void process(T value) { // Generic implementation std::cout << "Generic Processing: " << value << std::endl; } template <> void process<int>(int value) { // Specialized implementation for int type std::cout << "Special Processing for int: " << value << std::endl; }
In the above example, the `process` function template is defined with a generic implementation that can handle any type. However, an explicit specialization is provided for the `int` type. When the `process` function is called with an `int` argument, the specialized implementation for `int` will be used instead of the generic implementation.
2.Partial specialization:
Partial specialization allows you to provide a specialized implementation for a subset of types. It involves defining a template specialization with one or more template parameters partially specialized.
Here’s an example of partial specialization for a template class:
template <typename T, typename U> class Pair { public: Pair(T first, U second) : first(first), second(second) {} void display() { std::cout << "Generic Pair: " << first << ", " << second << std::endl; } }; template <typename T> class Pair<T, int> { public: Pair(T first, int second) : first(first), second(second) {} void display() { std::cout << "Partial Specialization for int: " << first << ", " << second << std::endl; } };
In the above example, the `Pair` class template is defined with two template parameters, `T` and `U`. The generic implementation of the class can work with any pair of types. However, a partial specialization is provided for the case where the second type is `int`. When the `Pair` class is instantiated with `T` and `int`, the partial specialization will be used, overriding the generic implementation.
Here’s an example usage of the specialized template functions and class:
int main() { process("Hello"); // Generic Processing: Hello process(10); // Special Processing for int: 10 Pair<double, char> pair1(3.14, 'A'); pair1.display(); // Generic Pair: 3.14, A Pair<int, int> pair2(5, 10); pair2.display(); // Partial Specialization for int: 5, 10 return 0; }
In the `main()` function, the specialized versions of the template functions and class are used based on the provided template arguments. The appropriate specialized implementation is selected at compile-time.
Template specialization provides a way to customize the behavior of template functions or classes for specific types, allowing you to optimize or adapt the implementation as needed.
5. Discuss the concept of template specialization in C++.
In C++, you can specialize a template function or class to provide a specific implementation for a particular type or set of types. Template specialization allows you to customize the behavior of a template when it is used with specific template arguments.
There are two types of template specialization: explicit specialization and partial specialization.
1. Explicit specialization:
Explicit specialization allows you to provide a specialized implementation for a specific type. It involves explicitly defining the template function or class for the desired type.
Here’s an example of explicit specialization for a template function:
template <typename T> void process(T value) { // Generic implementation std::cout << "Generic Processing: " << value << std::endl; } template <> void process<int>(int value) { // Specialized implementation for int type std::cout << "Special Processing for int: " << value << std::endl; }
In the above example, the `process` function template is defined with a generic implementation that can handle any type. However, an explicit specialization is provided for the `int` type. When the `process` function is called with an `int` argument, the specialized implementation for `int` will be used instead of the generic implementation.
2. Partial specialization:
Partial specialization allows you to provide a specialized implementation for a subset of types. It involves defining a template specialization with one or more template parameters partially specialized.
Here’s an example of partial specialization for a template class:
template <typename T, typename U> class Pair { public: Pair(T first, U second) : first(first), second(second) {} void display() { std::cout << "Generic Pair: " << first << ", " << second << std::endl; } }; template <typename T> class Pair<T, int> { public: Pair(T first, int second) : first(first), second(second) {} void display() { std::cout << "Partial Specialization for int: " << first << ", " << second << std::endl; } };
In the above example, the `Pair` class template is defined with two template parameters, `T` and `U`. The generic implementation of the class can work with any pair of types. However, a partial specialization is provided for the case where the second type is `int`. When the `Pair` class is instantiated with `T` and `int`, the partial specialization will be used, overriding the generic implementation.
Here’s an example usage of the specialized template functions and class:
int main() { process("Hello"); // Generic Processing: Hello process(10); // Special Processing for int: 10 Pair<double, char> pair1(3.14, 'A'); pair1.display(); // Generic Pair: 3.14, A Pair<int, int> pair2(5, 10); pair2.display(); // Partial Specialization for int: 5, 10 return 0; }
In the `main()` function, the specialized versions of the template functions and class are used based on the provided template arguments. The appropriate specialized implementation is selected at compile-time.
Template specialization provides a way to customize the behavior of template functions or classes for specific types, allowing you to optimize or adapt the implementation as needed.
6. Implement a template class for a generic stack data structure.
Certainly! Here’s an example of a template class implementation for a generic stack data structure:
template <typename T> class Stack { private: static const int MAX_SIZE = 100; // Maximum size of the stack T data[MAX_SIZE]; // Array to store stack elements int topIndex; // Index of the top element public: Stack() : topIndex(-1) {} bool isEmpty() const { return (topIndex == -1); } bool isFull() const { return (topIndex == MAX_SIZE - 1); } void push(const T& element) { if (isFull()) { std::cout << "Stack Overflow!" << std::endl; return; } data[++topIndex] = element; } void pop() { if (isEmpty()) { std::cout << "Stack Underflow!" << std::endl; return; } --topIndex; } T& top() { if (isEmpty()) { std::cout << "Stack is empty!" << std::endl; throw std::runtime_error("Stack is empty!"); } return data[topIndex]; } };
In the above example, a template class `Stack` is defined, which can be instantiated with any data type `T`. The private members of the class include a static constant `MAX_SIZE` to specify the maximum size of the stack, an array `data` to store the stack elements, and an `topIndex` to keep track of the top element’s index.
The public member functions of the class include:
- `Stack()` constructor: Initializes the stack by setting the `topIndex` to -1.
- `isEmpty()`: Checks if the stack is empty by comparing the `topIndex` with -1.
- `isFull()`: Checks if the stack is full by comparing the `topIndex` with `MAX_SIZE – 1`.
- `push(const T& element)`: Pushes an element onto the stack. If the stack is full, it displays an error message.
- `pop()`: Pops the top element from the stack. If the stack is empty, it displays an error message.
- `top()`: Returns a reference to the top element of the stack. If the stack is empty, it throws a `std::runtime_error` exception.
Here’s an example usage of the `Stack` class:
int main() { Stack<int> intStack; intStack.push(10); intStack.push(20); intStack.push(30); std::cout << "Top element of intStack: " << intStack.top() << std::endl; intStack.pop(); std::cout << "Top element of intStack after pop: " << intStack.top() << std::endl; Stack<std::string> stringStack; stringStack.push("Hello"); stringStack.push("World"); stringStack.push("!"); std::cout << "Top element of stringStack: " << stringStack.top() << std::endl; return 0; }
In the `main()` function, two instances of the `Stack` class are created: `intStack` with `int` as the template argument, and `stringStack` with `std::string` as the template argument. Elements are pushed onto the stacks using the `push()` function, and the top elements are retrieved using the `top()` function. The `pop()` function is used to remove the top element from `intStack`. The results are then printed to the console.
Output:
Top element of intStack: 30 Top element of intStack after pop: 20 Top element of stringStack: !
With this template class, you can create stack objects of different types and perform stack operations on them using a unified interface.
7. How can you restrict template parameters to specific types using type traits?
In C++, you can use type traits along with template metaprogramming techniques to restrict template parameters to specific types. Type traits are a set of template classes provided by the C++ standard library that provide compile-time information about types. By utilizing type traits, you can enable or disable template specializations based on certain conditions, such as checking if a type is a pointer, an integral type, or satisfying other requirements.
Here’s an example of how you can use type traits to restrict template parameters to specific types:
#include <type_traits> template <typename T> void process(T value) { static_assert(std::is_integral_v<T>, "T must be an integral type."); // Rest of the code for processing integral types... }
In the above example, the `process` function template takes a template parameter `T`. The `std::is_integral_v<T>` expression is used to check if `T` is an integral type using the `std::is_integral` type trait. If `T` is not an integral type, a static assertion will be triggered, and the compiler will generate an error message with the provided error message string.
You can apply various type traits to enforce restrictions on template parameters. Some commonly used type traits include `std::is_integral`, `std::is_floating_point`, `std::is_pointer`, `std::is_class`, `std::is_same`, and many more. These traits provide information about the properties of types, allowing you to conditionally enable or disable template specializations based on those properties.
Here’s another example that demonstrates how to restrict a template parameter to a specific class type:
#include <type_traits> class MyClass { // Class implementation... }; template <typename T> void process(T value) { static_assert(std::is_same_v<T, MyClass>, "T must be of type MyClass."); // Rest of the code for processing MyClass objects... }
In this example, the `process` function template ensures that the template parameter `T` must be of type `MyClass` using the `std::is_same` type trait. If `T` is not the same type as `MyClass`, a static assertion will be triggered.
By leveraging type traits, you can perform compile-time checks and constraints on template parameters, allowing you to restrict template instantiations to specific types and ensure the expected behavior of your template code.
8. Explain the difference between template classes and template functions.
In C++, templates can be used to create both template classes and template functions. While both template classes and template functions are used to generate generic code that can work with different types, there are some key differences between them.
Template Classes:
A template class is a blueprint for creating a family of classes. It allows you to define a class with generic types that are determined at the time of instantiation. The class template can have member variables, member functions, and member types that are parameterized by one or more template parameters.
Here are some key characteristics of template classes:
1. Type parameterization:
Template classes allow you to parameterize the class with one or more type parameters. These type parameters can be used to define member variables, member functions, and member types within the class.
2. Instance-specific behavior:
Each instance of a template class represents a specific version of the class specialized with the provided template arguments. Each instance can have its own set of member variables and member functions based on the provided types.
3. Multiple instantiations:
Template classes can be instantiated multiple times with different template arguments, resulting in multiple distinct class types. Each instantiation can have its own set of member variables and member functions based on the provided types.
Template Functions:
A template function is a function that is defined using a template. It allows you to write a single function definition that can operate on different types. The function template is a blueprint that is used to generate multiple versions of the function for different types. Here are some key characteristics of template functions:
1. Type parameterization:
Template functions allow you to parameterize the function with one or more type parameters. These type parameters can be used within the function’s code to define the types of function parameters, return types, and local variables.
2. Generic behavior:
A template function defines a generic behavior that is applied to multiple types. The function can operate on different types by substituting the template parameters with the provided types during function instantiation.
3. Single function definition:
The template function has a single function definition that can be used for different types. The compiler generates specialized versions of the function for each set of template arguments used during function calls.
In summary, template classes are used to define a family of classes that can be instantiated with different types, providing instance-specific behavior for each specialization. Template functions, on the other hand, allow you to define a single function that can operate on different types, generating specialized versions of the function for each set of template arguments. Both template classes and template functions provide a way to write generic code, but they differ in their scope and the way they generate multiple versions of the code.
9. Write a template function to calculate the average of an array of numbers.
Certainly! Here’s an example of a template function that calculates the average of an array of numbers:
#include <iostream>
template <typename T, size_t N> T calculateAverage(const T (&array)[N]) { T sum = 0; for (size_t i = 0; i < N; ++i) { sum += array[i]; } return sum / static_cast<T>(N); } int main() { int intArray[] = {1, 2, 3, 4, 5}; double doubleArray[] = {1.5, 2.5, 3.5, 4.5, 5.5}; std::cout << "Average of intArray: " << calculateAverage(intArray) << std::endl; std::cout << "Average of doubleArray: " << calculateAverage(doubleArray) << std::endl; return 0; }
In the above example, the `calculateAverage` function template takes an array `array` as a parameter, along with its size `N`. The template parameter `T` represents the type of the array elements. The function calculates the sum of all elements in the array and divides it by the size of the array to obtain the average.
In the `main()` function, two arrays `intArray` and `doubleArray` are declared and initialized with integer and double values, respectively. The `calculateAverage` function is called with each array, and the calculated average is printed to the console.
Output: Average of intArray: 3 Average of doubleArray: 3.5
With this template function, you can calculate the average of arrays of various types, such as integers, floating-point numbers, or other numeric types.
10. Discuss the concept of template metaprogramming and provide an example.
Template metaprogramming is a technique in C++ that leverages the power of templates to perform computations and generate code at compile-time. It allows you to perform complex operations, such as conditional branching, iteration, and type manipulation, during the compilation process.
Template metaprogramming exploits the template mechanism, template specialization, and the SFINAE (Substitution Failure Is Not An Error) principle to achieve compile-time computation.The key idea behind template metaprogramming is that templates can be instantiated with both types and non-type values. By exploiting this feature, you can define template classes and functions that can be evaluated and resolved at compile-time to perform computations, generate types, and even produce optimized code.
Here’s a simple example that demonstrates template metaprogramming to calculate the factorial of a given number at compile-time:
#include <iostream> template <int N> struct Factorial { static const int value = N * Factorial<N - 1>::value; }; template <> struct Factorial<0> { static const int value = 1; }; int main() { constexpr int number = 5; std::cout << "Factorial of " << number << ": " << Factorial<number>::value << std::endl; return 0; }
In the above example, the `Factorial` template struct is defined to calculate the factorial of a given number. The template struct recursively computes the factorial by defining a static constant `value` that holds the result.
The primary template `Factorial<N>` defines `value` as the product of `N` and `Factorial<N – 1>::value`. This recursive definition continues until `N` becomes 0.
The specialization `Factorial<0>` is provided as the base case, which defines `value` as 1. This is necessary to terminate the recursive computation.
In the `main()` function, the `Factorial<number>::value` expression calculates the factorial of the constant value `number` at compile-time. The result is printed to the console.
Output: Factorial of 5: 120
In this example, the template metaprogramming technique is used to calculate the factorial of a number at compile-time. The computation is performed during the compilation process, eliminating the runtime overhead.
Template metaprogramming enables powerful compile-time computations and code generation, allowing for optimizations, static checks, and flexibility in creating generic and efficient code. It can be used in various scenarios, such as type traits, static assertions, compile-time optimizations, and more.
Exceptions:
1. What are exceptions in C++? How are they useful?
Exceptions in C++ are a mechanism that allows for handling and propagating exceptional or error conditions that occur during program execution. They provide a structured way to handle errors and abnormal situations, making the code more robust and maintainable.
Exceptions are particularly useful in scenarios where error conditions cannot be handled locally and need to be propagated to higher levels of the program.
Here are some key aspects of exceptions in C++:
1. Exception Types:
Exceptions are objects that are thrown when an exceptional condition occurs. These exceptions can be of any type, including built-in types, user-defined types, or even class hierarchies.
2. Throwing Exceptions:
When an exceptional condition occurs, you can explicitly throw an exception using the `throw` keyword. The `throw` statement interrupts the normal flow of the program and transfers control to an exception handler.
3. Exception Handlers:
Exception handlers, also known as catch blocks, are used to handle thrown exceptions. They are written using the `try-catch` construct. A `try` block is used to enclose the code that may throw an exception, and a `catch` block is used to handle the thrown exception.
4. Exception Propagation:
If an exception is thrown inside a `try` block and there is no matching `catch` block within that block, the exception propagates up the call stack until it finds a suitable `catch` block. This allows for centralized error handling and separation of error-handling code from normal program logic.
5. Exception Safety:
Exceptions provide a mechanism for achieving exception safety, which involves ensuring that resources are properly released and the program remains in a consistent state even in the presence of exceptions. Exception safety is crucial for maintaining program integrity.
The use of exceptions in C++ brings several advantages:
- Error Handling: Exceptions provide a structured way to handle errors, allowing for cleaner and more readable code. Exception handling code can be separated from the normal program flow, making the code easier to understand and maintain.
- Centralized Error Handling: Exceptions allow for centralized error handling by propagating exceptions up the call stack until an appropriate handler is found. This helps in separating error handling logic from the core program logic, promoting modularity and code reuse.
- Graceful Recovery: Exceptions enable graceful recovery from exceptional conditions by providing the ability to catch and handle specific exceptions. This allows for taking appropriate actions, such as logging errors, notifying users, or attempting alternative approaches.
- Resource Cleanup: Exceptions support automatic cleanup of resources through destructors. When an exception is thrown, the destructors of objects in the call stack are called in reverse order, ensuring that resources are properly released, even if exceptions are thrown.
However, it’s important to note that exceptions should be used judiciously. They are most suitable for exceptional and error scenarios, rather than for expected or predictable conditions. Proper exception design and handling practices should be followed to ensure robust and reliable code.
2. How are exceptions thrown and caught in C++?
Exceptions in C++ are thrown using the `throw` keyword and caught using the `try-catch` construct.
Let’s look at how exceptions are thrown and caught in C++:
Throwing Exceptions:
Exceptions are thrown when an exceptional condition occurs during the execution of a program. To throw an exception, you use the `throw` keyword followed by an expression representing the exception object. The expression can be of any type, including built-in types, user-defined types, or even class hierarchies.
Here’s an example of throwing an exception:
void divide(int a, int b) { if (b == 0) { throw "Division by zero is not allowed!"; } int result = a / b; // ... }
In the above example, the `divide` function throws an exception of type `const char*` with the message “Division by zero is not allowed!” if the divisor `b` is zero.
Catching Exceptions:
To handle thrown exceptions, you use the `try-catch` construct. The `try` block encloses the code that may throw an exception, and the `catch` block(s) define the exception handlers that handle specific types of exceptions.
Here’s an example of catching an exception:
int main() { try { divide(10, 0); } catch (const char* error) { std::cout << "Exception caught: " << error << std::endl; } // ... return 0; }
In the above example, the `divide` function is called within a `try` block. If an exception is thrown inside the `divide` function (in case of division by zero), the corresponding `catch` block is executed. The `catch` block catches the exception of type `const char*` and displays the error message.
Multiple `catch` blocks can be used to handle different types of exceptions. The exception is matched against each `catch` block in the order they appear, and the first matching `catch` block is executed. If no matching `catch` block is found, the exception propagates up the call stack until a suitable handler is found.
Exception Handling Hierarchy:
In addition to handling specific exceptions, you can also catch exceptions based on their base types. This allows for handling a group of related exceptions in a single `catch` block. By catching exceptions based on their base types, you can handle exceptions hierarchically.
Here’s an example of catching exceptions based on a common base type:
class BaseException { /* Base class for exceptions */ }; class DerivedException : public BaseException { /* Derived class */ }; void foo() { if (/* some condition */) { throw DerivedException(); } // ... } int main() { try { foo(); } catch (BaseException& ex) { std::cout << "BaseException caught!" << std::endl; } // ... return 0; }
In this example, the `foo` function may throw an exception of type `DerivedException`. However, the `catch` block in the `main` function catches the exception based on its base type `BaseException`. This allows for handling exceptions of the derived type as well as any other exceptions derived from `BaseException`.
By using the `try-catch` construct, you can effectively handle thrown exceptions, allowing for proper error handling, graceful recovery, and resource cleanup in your C++ programs.
3. Explain the difference between try-catch and throw in exception handling.
In C++, exception handling involves two main components: `try-catch` and `throw`.
Let’s discuss the difference between these two components:
try-catch:
The `try-catch` construct is used to handle exceptions. It allows you to specify a block of code where exceptions might be thrown (`try` block) and define one or more blocks of code to handle those exceptions (`catch` blocks).
- The `try` block contains the code that may potentially throw an exception. It is enclosed within the `try` keyword followed by a pair of curly braces. If an exception is thrown within the `try` block, the normal flow of execution is interrupted, and the program looks for a matching `catch` block to handle the exception.
- The `catch` block is used to catch and handle exceptions thrown within the associated `try` block. It is written using the `catch` keyword followed by a parameter declaration in parentheses and a block of code enclosed within curly braces.
The parameter declaration specifies the type of exception that can be caught and provides a name for the exception object that will hold the thrown exception. If the type of the thrown exception matches the type specified in the `catch` block, the corresponding `catch` block is executed.
Here’s an example that demonstrates the `try-catch` construct:
try { // Code that may throw exceptions // ... } catch (ExceptionType1& ex1) { // Exception handler for ExceptionType1 // ... } catch (ExceptionType2& ex2) { // Exception handler for ExceptionType2 // ... } catch (...) { // Default exception handler // ... }
In this example, the code within the `try` block can throw exceptions of different types. Each `catch` block is associated with a specific exception type and defines the corresponding exception handler. The last `catch` block with `…` is a catch-all block that handles any other exceptions that do not match the types specified in the preceding `catch` blocks.
throw:
The `throw` keyword is used to explicitly throw an exception when an exceptional condition occurs during the execution of a program. When an exception is thrown, it interrupts the normal flow of execution and transfers control to an appropriate `catch` block.
- The `throw` statement is written using the `throw` keyword followed by an expression representing the exception object that is being thrown. The expression can be of any type, including built-in types, user-defined types, or even class hierarchies.
Here’s an example of throwing an exception:
if (condition) { throw ExceptionType("Error message"); }
In this example, the `throw` statement is used to throw an exception of type `ExceptionType` with the specified error message when a certain condition is met.
To summarize, the `try-catch` construct provides a way to handle exceptions by enclosing the code that might throw exceptions within a `try` block and defining `catch` blocks to handle those exceptions. On the other hand, the `throw` keyword is used to explicitly throw an exception when an exceptional condition is encountered. The `try-catch` construct catches and handles the thrown exceptions based on their types.
4. Write a program that demonstrates the use of exception handling in file I/O operations.
Certainly! Here’s an example program that demonstrates the use of exception handling in file I/O operations in C++:
#include <iostream> #include <fstream>
int main() { std::ifstream inputFile; std::string filename; std::cout << "Enter the filename: "; std::cin >> filename; try { inputFile.open(filename); if (!inputFile.is_open()) { throw std::runtime_error("Failed to open the file."); } std::string line; while (std::getline(inputFile, line)) { std::cout << line << std::endl; } inputFile.close(); } catch (const std::exception& e) { std::cerr << "Exception: " << e.what() << std::endl; } return 0; }
In this program, we open a file specified by the user, read its contents, and display them on the console. Exception handling is used to handle any potential errors that may occur during the file I/O operations.
Here’s how the program works:
- The program prompts the user to enter a filename.
- The filename entered by the user is stored in the `filename` variable.
- Inside the `try` block, we attempt to open the file using the `open` method of `std::ifstream`.
- If the file fails to open (i.e., `inputFile.is_open()` returns `false`), we throw a `std::runtime_error` exception with a descriptive error message.
- If the file is successfully opened, we read its contents line by line using `std::getline` and display each line on the console.
- Finally, we close the file using `inputFile.close()`.
- If any exception occurs during the `try` block, it is caught in the `catch` block. The caught exception is of type `const std::exception&`, which is the base class for all standard exceptions. The error message associated with the exception is displayed on the standard error stream (`std::cerr`).
By using exception handling in file I/O operations, we can gracefully handle errors such as file not found, permission issues, or any other I/O-related errors that may occur during the program’s execution.
5. What happens if an exception is not caught in C++?
If an exception is not caught in C++, it will result in program termination. When an exception is thrown but not caught by any `catch` block, it triggers a mechanism called “stack unwinding” where the program’s execution stack is unwound, and the program terminates abruptly.
Here’s what happens when an exception is not caught:
1. The exception is thrown: When an exception is thrown, the program looks for a matching `catch` block to handle the exception. It starts searching for the appropriate `catch` block in the current scope. If a matching `catch` block is found, the program jumps to that `catch` block, and the exception is considered handled. However, if no matching `catch` block is found in the current scope, the program moves to the next outer scope and continues the search.
2. Stack unwinding: If the program fails to find a matching `catch` block in any of the scopes, the exception remains unhandled, and the stack unwinding process begins. During stack unwinding, the program unwinds the call stack, executing the destructors of objects in reverse order of their construction. The stack unwinding process continues until an appropriate `catch` block is found or until the stack is completely unwound.
3. Termination: If the stack is completely unwound without finding a suitable `catch` block, the program terminates. The termination can be abrupt, and the exact behavior may vary depending on the platform and compiler. Typically, an uncaught exception leads to program termination with an error message indicating the unhandled exception type and a stack trace.It is generally recommended to handle exceptions appropriately in your code to ensure graceful error handling, proper cleanup, and prevent unexpected program termination.
By catching exceptions and handling them in a controlled manner, you can provide meaningful error messages to the user, perform necessary cleanup operations, and potentially recover from exceptional conditions.
6. Discuss the concept of exception specifications and their usage.
In C++, exception specifications are a feature that allows you to specify the types of exceptions that a function may throw. Exception specifications provide a way to document and enforce the exception safety guarantees of a function.
However, it’s important to note that exception specifications have been deprecated in C++11 and are no longer recommended for use. Instead, C++11 introduced the concept of exception handling based on `try-catch` blocks, which provides a more flexible and robust approach to handling exceptions.Prior to C++11, exception specifications were declared using the `throw` keyword followed by a comma-separated list of exception types inside parentheses, as part of the function declaration. For example:
void myFunction() throw (ExceptionType1, ExceptionType2);
The exception specification indicates that the function `myFunction` is expected to throw exceptions of types `ExceptionType1` and `ExceptionType2`. If any other type of exception is thrown from the function that is not specified in the exception specification, the `std::unexpected` function is called, which by default leads to `std::terminate` being called and the program being terminated.
However, exception specifications have several limitations and drawbacks:
1. Limited Expressiveness: Exception specifications can only specify a list of exception types but do not provide any information about the specific conditions or reasons for throwing exceptions.
2. No Enforcement: Exception specifications are not enforced at runtime. If a function throws an exception that is not specified in its exception specification, the behavior is undefined. It means that exception specifications do not provide compile-time or runtime checks to ensure that the function adheres to the specified exception types.
3. Unnecessary Restriction: Exception specifications can unnecessarily restrict the types of exceptions that a function can throw. It may hinder code reuse and prevent the use of more generic exception handling mechanisms.
As a result of these limitations, exception specifications were deprecated in C++11, and the focus shifted towards using `try-catch` blocks for handling exceptions. `try-catch` blocks provide a more flexible and expressive way to handle exceptions and allow for more fine-grained control over exception handling and recovery.
In modern C++, it is recommended to use `try-catch` blocks for handling exceptions and to document the potential exceptions thrown by a function using comments, documentation, or other appropriate means.
7. How can you create your own custom exception classes in C++?
In C++, you can create your own custom exception classes by deriving them from the standard exception class or its derived classes.
Here’s an example of creating a custom exception class:
#include <iostream> #include <exception> class MyException : public std::exception { public: MyException(const char* message) : std::exception(message) {} }; int main() { try { throw MyException("Custom exception occurred"); } catch (const MyException& e) { std::cout << "Caught exception: " << e.what() << std::endl; } return 0; }
In this example, we define a custom exception class called `MyException` by inheriting from the `std::exception` base class. The `std::exception` class provides a standard interface for exceptions and includes a member function `what()` that returns a C-style string describing the exception.
Inside the `MyException` class, we define a constructor that takes a `const char*` parameter representing the exception message. We pass this message to the base class constructor of `std::exception` using the initializer list.
In the `main()` function, we demonstrate throwing and catching the custom exception. We use a `try-catch` block to catch the exception of type `MyException`. When the exception is thrown, it is caught by the corresponding `catch` block, and the `what()` function is called to retrieve and display the exception message.
You can further customize your custom exception class by adding additional member variables or member functions as per your requirements. It is recommended to provide meaningful information and context in the exception message to aid in debugging and error handling.
By creating custom exception classes, you can define your own exception hierarchy and handle specific exceptional conditions in a more tailored and meaningful way in your code.
8. Explain the concept of stack unwinding in relation to exceptions.
In the context of exception handling, stack unwinding is a process that occurs when an exception is thrown and not immediately caught by a matching `catch` block. Stack unwinding involves the unwinding of the call stack, which means that the program jumps out of nested function calls, executing the necessary cleanup operations along the way.
Here’s how stack unwinding works:
1. Exception is thrown: When an exception is thrown, the program starts searching for a suitable `catch` block to handle the exception. It begins the search in the current scope and proceeds to outer scopes until a matching `catch` block is found.
2. Stack unwinding starts: If the program fails to find a matching `catch` block in the current scope or any outer scope, the stack unwinding process begins. The stack is unwound by unwinding the function call stack in reverse order of function calls. This means that the functions that were called most recently are unwound first, followed by their parent functions and so on.
3. Destructor execution: As the stack unwinding progresses, the destructors of the objects in the unwound function calls are invoked. This allows the objects to perform necessary cleanup operations, such as releasing resources, closing files, deallocating memory, and restoring invariants.
4. Stack continues unwinding: The stack unwinding process continues until a suitable `catch` block is found or until the stack is completely unwound. If a matching `catch` block is found, the program transfers control to that `catch` block, and the exception is considered handled. If the stack is completely unwound without finding a matching `catch` block, the program terminates.
The purpose of stack unwinding is to ensure that all necessary cleanup operations are performed before the program terminates due to an unhandled exception. This helps in maintaining the integrity of the program’s state and preventing resource leaks or other undesirable side effects.
It’s important to note that during stack unwinding, the destructors of objects in the unwound stack frames are called automatically, even if they were not explicitly called in the code. This automatic invocation of destructors ensures that objects are properly cleaned up, regardless of whether an exception occurred or not.
By properly designing and implementing destructors, you can ensure that necessary cleanup operations are performed reliably and consistently, even in the presence of exceptions.
9. How does exception handling differ in C++ compared to other programming languages?
Exception handling in C++ differs from other programming languages in a few key aspects.
Here are some notable differences:
1. Syntax: The syntax for exception handling in C++ involves the use of `try`, `catch`, and `throw` keywords. The `try` block encloses the code that may potentially throw an exception, and the `catch` block is used to handle the thrown exception. Exceptions are explicitly thrown using the `throw` keyword. Other programming languages may have different syntax or keywords for exception handling.
2. Strongly Typed Exceptions: C++ supports strongly typed exceptions, meaning that exceptions are associated with specific types. This allows for more precise exception handling based on the type of exception thrown. In contrast, some languages have more loosely typed or untyped exceptions, where exception objects may not have specific types or are handled more generically.
3. Exception Hierarchies: C++ allows for the creation of custom exception classes by deriving from standard exception classes or their derived classes. This enables the creation of an exception hierarchy, where different types of exceptions can be caught and handled separately based on their derived classes. Some programming languages may have a simpler exception model without explicit support for exception hierarchies.
4. Resource Management: Exception handling in C++ is often used in conjunction with resource management techniques, such as RAII (Resource Acquisition Is Initialization). With RAII, resources are acquired during object construction and released in the object’s destructor. Exceptions can be used to ensure proper resource cleanup by allowing destructors to be called automatically during stack unwinding. Not all languages have the same level of support for deterministic resource cleanup tied to exception handling.
5. Performance Considerations: Exception handling in C++ may have performance implications, especially when exceptions are thrown and caught frequently. The overhead of constructing exception objects, stack unwinding, and searching for matching `catch` blocks can impact performance. In contrast, some languages may have alternative error handling mechanisms, such as return codes or optional types, that may have different performance characteristics.
It’s important to note that while exception handling is a powerful and widely used technique in C++, it may not be the preferred or only mechanism for error handling in all programming languages. Different languages have different philosophies and approaches to error handling, and the choice of exception handling or alternative mechanisms depends on the language’s design principles, programming paradigms, and performance considerations.
10. Discuss the advantages and disadvantages of using exceptions in C++.
Exceptions in C++ provide a powerful mechanism for handling exceptional conditions and errors in a structured and controlled manner. However, like any language feature, exceptions have their own advantages and disadvantages.
Let’s discuss them:Advantages of Exceptions in C++:
1. Separation of Error Handling Code: Exceptions allow for the separation of error handling code from normal code. This promotes cleaner code organization by keeping error handling logic separate from the main logic, leading to better code readability and maintainability.
2. Centralized Error Handling: Exceptions provide a centralized and uniform mechanism for handling errors and exceptional conditions. With exceptions, error handling code can be consolidated in `catch` blocks, making it easier to manage and update error handling logic.
3. Stack Unwinding and Resource Cleanup: Exceptions trigger stack unwinding, which ensures that destructors are called along the way. This enables automatic and reliable cleanup of resources, such as closing files, releasing memory, and releasing locks, even in the presence of exceptions. This promotes better resource management and reduces the risk of resource leaks.
4. Error Propagation: Exceptions allow for error propagation through the call stack. When an exception is thrown, it can be caught at different levels of the call stack, providing an opportunity to handle the error at an appropriate level. This simplifies error handling and allows for more flexible error recovery strategies.
Disadvantages of Exceptions in C++:
1. Performance Overhead: Exception handling can introduce performance overhead, especially when exceptions are frequently thrown and caught. The overhead includes the cost of constructing and destructing exception objects, stack unwinding, and searching for appropriate `catch` blocks. In performance-critical scenarios, the use of exceptions needs to be carefully considered.
2. Code Complexity: Exception handling can increase code complexity. Exception-safe code requires careful design and implementation of exception handling mechanisms, such as handling resource cleanup, preserving program state, and maintaining invariants. Handling exceptions in a consistent and correct manner may require additional effort and may complicate the code structure.
3. Potential for Misuse: Exceptions can be misused or overused, leading to code that is difficult to understand and maintain. If exceptions are used for non-exceptional control flow or as a replacement for regular error handling, it can result in convoluted code and hinder code readability.
4. Portability and Compatibility: Exception handling mechanisms may have variations across different compilers and platforms, which can affect code portability. Additionally, some programming environments or embedded systems may have limited support for exceptions, making their usage less viable or not recommended.
It’s important to use exceptions judiciously, considering the specific requirements and constraints of the application. Exception handling should be used for exceptional and exceptional error conditions, where it adds value in terms of code organization, error recovery, and resource management.
Careful consideration should be given to performance implications and the impact on code complexity when deciding to use exceptions in C++.
STL (Standard Template Library):
1. What is the Standard Template Library (STL) in C++?
The Standard Template Library (STL) in C++ is a library that provides a collection of generic algorithms and data structures. It is part of the C++ Standard Library and is widely used for its rich set of container classes, algorithms, and function objects.The STL is designed to be efficient, flexible, and reusable, offering a high-level abstraction for common programming tasks.
It is based on the principles of generic programming, allowing algorithms and data structures to be implemented in a way that is independent of the underlying data types.
Key components of the STL include:
- Containers: The STL provides various container classes, such as vectors, lists, queues, stacks, sets, maps, and more. These containers offer different characteristics and functionality to suit specific requirements.
- Algorithms: The STL includes a large collection of algorithms that operate on the container elements. These algorithms cover a wide range of operations, including sorting, searching, transforming, manipulating, and merging data.
- Iterators: Iterators are used to access and traverse elements within containers. The STL provides different types of iterators, such as input iterators, output iterators, forward iterators, bidirectional iterators, and random access iterators. They provide a uniform interface for accessing container elements, allowing algorithms to work seamlessly with different containers.
- Function Objects: Function objects (also known as functors) are objects that act like functions. The STL utilizes function objects extensively, providing predefined function objects for common operations. Additionally, users can define their own function objects to customize algorithm behavior.
The STL promotes code reuse, algorithmic clarity, and efficiency by providing well-designed generic components. It follows the principle of “don’t reinvent the wheel” by offering a rich set of ready-to-use algorithms and containers, allowing developers to focus on solving higher-level problems rather than implementing low-level data structures and algorithms from scratch.
By leveraging the STL, C++ developers can write code that is efficient, readable, and maintainable, while benefiting from a standardized and widely-used library.
2. Discuss the three main components of the STL.
The Standard Template Library (STL) in C++ consists of three main components: containers, algorithms, and iterators.
Let’s discuss each component in more detail:
1. Containers:
Containers are classes that hold and manage collections of objects or values. They provide various data structures with different characteristics and usage patterns.
Some commonly used container classes in the STL include:
- Sequence Containers: These containers maintain the order of elements as they are inserted. Examples include `vector`, `list`, `deque`, `array`, and `forward_list`.
- Associative Containers: These containers organize elements in a sorted manner based on a specific sorting criterion. Examples include `set`, `multiset`, `map`, and `multimap`.
- Container Adapters: These containers provide a specific interface for accessing elements. Examples include `stack`, `queue`, and `priority_queue`.
Containers in the STL provide a consistent interface and operations for accessing and manipulating elements. They handle memory allocation and deallocation internally, making it convenient and efficient to work with collections of objects.
2. Algorithms:
Algorithms in the STL are generic functions that operate on containers or sequences of elements. They provide a wide range of operations, including searching, sorting, manipulating, transforming, and iterating over elements.
Some commonly used algorithms in the STL include:
- Sorting Algorithms: Algorithms like `sort`, `stable_sort`, and `partial_sort` for sorting elements based on a specific criterion.
- Searching Algorithms: Algorithms like `find`, `binary_search`, and `lower_bound` for searching elements within a container.
- Numeric Algorithms: Algorithms like `accumulate`, `transform`, and `inner_product` for performing mathematical operations on elements.
Algorithms in the STL are designed to work with different container types and can be used interchangeably. They are implemented in a generic and efficient manner, providing consistent behavior across different container types.
3. Iterators:
Iterators are used to access and traverse elements within containers. They provide a uniform interface for accessing elements in a container, allowing algorithms to work independently of the specific container type. Iterators act as generalized pointers, providing operations like dereferencing, incrementing, and decrementing.
The STL provides different types of iterators to cater to various needs, including input iterators, output iterators, forward iterators, bidirectional iterators, and random access iterators. Each iterator type offers a specific set of operations and capabilities, depending on the characteristics of the associated container.
Iterators play a crucial role in connecting algorithms with containers. Algorithms operate on ranges defined by iterators, allowing for generic and flexible manipulation of elements.
By combining these three components – containers, algorithms, and iterators – the STL provides a comprehensive and powerful toolkit for C++ developers. The components work together seamlessly, enabling efficient, reusable, and generic code that promotes code clarity, productivity, and maintainability.
3. Explain the difference between containers, algorithms, and iterators in the STL.
In the context of the Standard Template Library (STL) in C++, containers, algorithms, and iterators are three distinct components that work together to provide a powerful and flexible framework for working with data.
Here’s an explanation of the differences between these components:
1. Containers:
Containers in the STL are classes that hold and manage collections of objects or values. They provide the underlying data structures for storing and organizing data. Containers define how the data is stored, accessed, and modified. Some common container classes in the STL include `vector`, `list`, `set`, `map`, and more. Containers manage memory allocation and deallocation internally, making it convenient for developers to work with collections of objects without worrying about memory management details.
2. Algorithms:
Algorithms in the STL are generic functions that operate on elements of containers or sequences. They perform various operations on the data contained within the containers. Algorithms can perform tasks such as searching, sorting, transforming, and manipulating data. Examples of algorithms in the STL include `find`, `sort`, `transform`, `count`, and many more. Algorithms are designed to be generic, meaning they can work with different container types. They operate on ranges defined by iterators and provide consistent behavior regardless of the specific container being used.
3. Iterators:
Iterators in the STL are objects that provide a way to access and traverse the elements within a container. They act as generalized pointers, allowing algorithms to iterate over the elements in a container. Iterators provide a uniform interface for accessing elements, regardless of the specific container type. They allow algorithms to work independently of the underlying container implementation. Iterators offer operations such as dereferencing, incrementing, decrementing, and comparison.
The STL provides various types of iterators, including input iterators, output iterators, forward iterators, bidirectional iterators, and random access iterators. Each iterator type provides a specific set of capabilities and operations based on the characteristics of the associated container.
In summary, containers are data structures that hold and manage collections of objects, algorithms are generic functions that perform operations on the elements within containers, and iterators provide a way to access and traverse the elements within containers. Together, these components provide a powerful and flexible framework for working with data in a generic and reusable manner.
4. Implement a stack using the stack container from the STL.
Certainly! Here’s an example of implementing a stack using the `std::stack` container from the STL in C++:
#include <iostream> #include <stack> int main() { std::stack<int> myStack; // Push elements onto the stack myStack.push(10); myStack.push(20); myStack.push(30); // Get the top element std::cout << "Top element: " << myStack.top() << std::endl; // Pop elements from the stack myStack.pop(); myStack.pop(); // Check if the stack is empty if (myStack.empty()) { std::cout << "Stack is empty" << std::endl; } else { std::cout << "Stack is not empty" << std::endl; } return 0; }
In this example, we include the necessary headers for `iostream` and `stack`. We create a stack called `myStack` using the `std::stack` template class. We then push elements onto the stack using the `push()` method. The `top()` method is used to retrieve the top element of the stack. We pop elements from the stack using the `pop()` method. Finally, we check if the stack is empty using the `empty()` method.
This implementation demonstrates the basic usage of the `std::stack` container from the STL for stack operations. You can add more elements, perform additional operations, or use the stack in your desired context.
5. How can you sort elements in a vector using the sort algorithm from the STL?
To sort elements in a vector using the `sort` algorithm from the STL in C++, follow these steps:
1. Include the necessary header for the `sort` algorithm:
#include <algorithm>
2. Create a vector and populate it with elements:
#include <iostream> #include <vector> int main() { std::vector<int> myVector = {5, 2, 8, 1, 9, 3}; // Or you can add elements one by one using push_back(): // std::vector<int> myVector; // myVector.push_back(5); // myVector.push_back(2); // ... // Output the vector before sorting std::cout << "Before sorting: "; for (int num : myVector) { std::cout << num << " "; } std::cout << std::endl;
3. Use the `std::sort` function to sort the vector. It takes two iterators representing the range to be sorted (in this case, the beginning and end of the vector). It rearranges the elements in ascending order by default:
// Sort the vector std::sort(myVector.begin(), myVector.end());
4. Output the vector after sorting:
// Output the vector after sorting std::cout << "After sorting: "; for (int num : myVector) { std::cout << num << " "; } std::cout << std::endl; return 0; }
When you run this program, it will output the vector before sorting and after sorting, demonstrating that the elements have been sorted in ascending order.
Note that the `std::sort` algorithm works with any type of container that provides random access iterators, including vectors. You can also specify a custom comparison function or use lambda expressions to define a specific sorting order.
// Sort in descending order using a custom comparison function std::sort(myVector.begin(), myVector.end(), [](int a, int b) { return a > b; });
With the `std::sort` algorithm from the STL, you have the flexibility to sort elements in a vector or any other container easily and efficiently.
6. Discuss the various types of iterators available in the STL.
In the Standard Template Library (STL) in C++, there are several types of iterators available. Each iterator type offers a different set of capabilities and operations based on the characteristics of the associated container.
Here are the main types of iterators in the STL:
1. Input Iterators:
- Characteristics: Read-only, single-pass, supports forward traversal.
- Capabilities: Can be dereferenced to read the value, can be incremented to move to the next element.
- Example Usage: `std::istream_iterator`, used for reading input from streams.
2. Output Iterators:
- Characteristics: Write-only, single-pass, supports forward traversal.
- Capabilities: Can be dereferenced to write the value, can be incremented to move to the next position.
- Example Usage: `std::ostream_iterator`, used for writing output to streams.
3. Forward Iterators:
- Characteristics: Read-write, multi-pass, supports forward traversal.
- Capabilities: Supports all operations of input iterators, can be compared for equality and inequality.
- Example Usage: `std::forward_list`, `std::unordered_set`.
4. Bidirectional Iterators:
- Characteristics: Read-write, multi-pass, supports bidirectional traversal (forward and backward).
- Capabilities: Supports all operations of forward iterators, can be decremented to move to the previous element.
- Example Usage: `std::list`, `std::set`, `std::map`.
5. Random Access Iterators:
- Characteristics: Read-write, multi-pass, supports random access traversal.
- Capabilities: Supports all operations of bidirectional iterators, can be incremented and decremented by arbitrary amounts, supports arithmetic operations like addition and subtraction, supports comparison operators.
- Example Usage: `std::vector`, `std::deque`, `std::array`.
The iterator categories listed above form a hierarchy, with each category providing a superset of capabilities compared to the previous category. For example, random access iterators provide all the capabilities of bidirectional iterators, which in turn provide all the capabilities of forward iterators, and so on.
It’s worth noting that iterators allow algorithms to operate on container elements in a generic and flexible manner. Algorithms can work with different container types as long as the appropriate iterator category is used.
By understanding and utilizing the various iterator types provided by the STL, you can effectively traverse, manipulate, and interact with the elements of different containers in a consistent and efficient manner.
7. How can you use the map container from the STL to implement a dictionary?
The `std::map` container from the STL in C++ is an associative container that allows you to implement a dictionary-like data structure.
Here’s an example of how you can use the `std::map` container to implement a dictionary:`
#include <iostream> #include <map> #include <string> int main() { std::map<std::string, std::string> dictionary; // Add entries to the dictionary dictionary["apple"] = "a round fruit with red or green skin"; dictionary["banana"] = "a long curved fruit with yellow skin"; dictionary["orange"] = "a round fruit with orange skin"; // Access and print definitions std::cout << "Dictionary entries:" << std::endl; std::cout << "apple: " << dictionary["apple"] << std::endl; std::cout << "banana: " << dictionary["banana"] << std::endl; std::cout << "orange: " << dictionary["orange"] << std::endl; // Check if a word exists in the dictionary std::string word; std::cout << "Enter a word to search in the dictionary: "; std::cin >> word; if (dictionary.count(word) > 0) { std::cout << "Definition: " << dictionary[word] << std::endl; } else { std::cout << "Word not found in the dictionary." << std::endl; } return 0; }
In this example, we include the necessary headers for `iostream`, `map`, and `string`. We create a `std::map` called `dictionary`, where the keys are words and the values are their corresponding definitions.
We add entries to the dictionary using the `[]` operator, where the key is the word and the value is its definition. We can access and print the definitions by using the keys as indices.
We then prompt the user to enter a word to search in the dictionary. We check if the word exists in the dictionary using the `count()` function, which returns the number of occurrences of a key. If the word exists, we print its definition; otherwise, we display a message indicating that the word was not found.
By using the `std::map` container, you can implement a dictionary-like structure that provides efficient search and retrieval of values based on keys. The container automatically maintains the keys in sorted order, allowing for fast lookup using binary search.
8. Explain the concept of functors (function objects) in the context of the STL.
In the context of the Standard Template Library (STL) in C++, functors, also known as function objects, are objects that behave like functions. They are instances of classes or structs that overload the function call operator `operator()`.
Functors provide a way to encapsulate and use function-like behavior as objects, allowing them to be passed as arguments to algorithms and used in generic programming. They are a powerful tool in the STL because they enable customization and flexibility in algorithm behavior.
Here are a few key points about functors in the STL:
1. Function-Like Behavior: Functors are designed to emulate the behavior of functions. They can be called with parentheses, just like functions, to perform a specific action or computation.
2. Customization: Functors allow you to customize the behavior of algorithms. By defining your own functor or using existing functors provided by the STL, you can modify how an algorithm operates on elements.
3. Stateful: Functors can maintain internal state, allowing them to remember information between calls. This state can be initialized in the constructor and updated during the execution of the functor.
4. Predicates and Comparators: Functors are commonly used as predicates in algorithms like `std::find_if` or as comparators in sorting algorithms like `std::sort`. They enable flexible conditions or custom sorting orders to be applied.
5. Greater Flexibility: Compared to regular function pointers, functors offer greater flexibility because they can have their own member variables and member functions. This makes them versatile for complex algorithms that require additional data or behavior.
Using functors in the STL allows you to leverage the power of object-oriented programming and functional programming paradigms. They provide a unified interface for algorithms to work with various types of operations, predicates, and custom behavior. Functors are an essential part of the STL’s generic programming model, enabling reusable and customizable components for working with containers and algorithms.
9. Write a program that demonstrates the usage of the STL’s list container.
Certainly! Here’s an example program that demonstrates the usage of the STL’s `std::list` container in C++:
#include <iostream> #include <list>
int main() {
std::list<int> myList;
// Add elements to the list
myList.push_back(10);
myList.push_back(20); myList.push_back(30); myList.push_front(5); // Print the elements of the list std::cout << "List elements: "; for (int num : myList) { std::cout << num << " "; } std::cout << std::endl; // Accessing elements by index (not available in std::list) // std::cout << "Element at index 1: " << myList[1] << std::endl; // Insert an element at a specific position std::list<int>::iterator it = std::next(myList.begin(), 2); myList.insert(it, 15); // Remove an element from the list myList.pop_back(); // Print the updated list std::cout << "Updated list elements: "; for (int num : myList) { std::cout << num << " "; } std::cout << std::endl; // Reverse the list myList.reverse(); // Print the reversed list std::cout << "Reversed list elements: "; for (int num : myList) { std::cout << num << " "; } std::cout << std::endl; // Clear the list myList.clear(); // Check if the list is empty if (myList.empty()) { std::cout << "The list is empty." << std::endl; } return 0; } ```
In this example, we include the necessary headers for `iostream` and `list`. We create a `std::list` called `myList` to store integers.
We add elements to the list using `push_back` and `push_front` to demonstrate adding elements at both ends. We then iterate over the list to print its elements.
Next, we demonstrate inserting an element at a specific position in the list using the `insert` function. We remove an element from the list using `pop_back`.
We then print the updated list and proceed to reverse its order using the `reverse` function. Finally, we clear the list and check if it is empty using the `empty` function.
Note that unlike other containers like `std::vector`, `std::list` does not support direct access to elements by index (e.g., `myList[1]`). To access elements, you can use iterators or functions like `std::next` to advance an iterator to a specific position.
When you run this program, it will demonstrate the basic operations of adding, inserting, removing, and iterating over elements in a `std::list`.
10. Discuss the advantages of using the STL in C++ programming.
Using the Standard Template Library (STL) in C++ programming offers several advantages that contribute to efficient and effective development.
Here are some key advantages of using the STL:
1. Code Reusability: The STL provides a collection of robust and reusable components like containers, algorithms, and iterators. These components are thoroughly tested, optimized, and designed to work together seamlessly. By utilizing the STL, you can leverage these pre-implemented components, reducing the need to reinvent the wheel and saving development time and effort.
2. Efficient and Performant: The STL is designed with performance in mind. The algorithms and containers in the STL are implemented using efficient data structures and optimized algorithms. They have been extensively tested and fine-tuned to provide high performance for common operations. Using the STL allows you to benefit from this optimization without having to implement your own algorithms or data structures.
3. Generic Programming: The STL promotes generic programming, which means writing code that is reusable across different data types. The STL components, such as containers and algorithms, are designed to work with any type that satisfies certain requirements (e.g., providing specific member functions). This generic approach allows you to write flexible and versatile code that can handle various data types without sacrificing performance.
4. Standardized Interface: The STL provides a standardized interface for common operations on containers and algorithms. This uniform interface allows developers to learn and understand the common functionality provided by the STL, making code more readable, maintainable, and easier to collaborate on. It also helps in reducing errors and improving code quality.
5. Safety and Robustness: The STL provides several safety mechanisms to prevent common programming errors. For example, iterators in the STL provide bounds checking to prevent accessing elements out of range, and algorithms often perform sanity checks on their input. Additionally, the use of templates in the STL provides type safety and compile-time checks, reducing the likelihood of runtime errors.
6. Community Support and Resources: The STL is widely used and has a large community of developers. This means that there are abundant resources, tutorials, and documentation available, making it easier to learn and troubleshoot issues. The STL is also well-integrated with the C++ language, and new features and improvements are continuously added based on community feedback and standards updates.
By leveraging the advantages of the STL, you can write efficient, reusable, and maintainable code. The STL components provide a solid foundation for handling common programming tasks, allowing you to focus on solving the specific problem at hand rather than reinventing common functionality.