OOP Using C++ – C++ Programming Inheritance based Questions and Answers
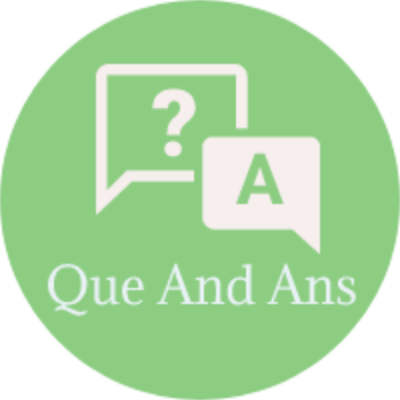
- Certainly! Here’s a list of 100 C++ programming inheritance-based questions for practicing inheritance concepts:
1. What is inheritance in C++?
Inheritance is a fundamental concept in object-oriented programming that allows a class to inherit properties (member variables and member functions) from another class. The class that inherits is called the “derived class” or “subclass,” and the class being inherited from is called the “base class” or “superclass.” Inheritance establishes a hierarchical relationship between classes, where the derived class inherits the characteristics of the base class.
2. What is the purpose of inheritance in object-oriented programming?
The purpose of inheritance in object-oriented programming is to promote code reuse and create a hierarchical structure of classes. It allows the derived class to inherit the properties and behaviors of the base class, reducing code duplication and improving maintainability. Inheritance also enables polymorphism, where objects of the derived class can be treated as objects of the base class, providing flexibility and extensibility in the design of software systems.
3. How do you declare a derived class in C++?
To declare a derived class in C++, you use the `class` keyword followed by the name of the derived class and the keyword `: public` followed by the base class from which it inherits. Here’s an example:
```cpp class DerivedClassName : public BaseClassName { // Member variables and member functions of the derived class }; ```
4. What is a base class in C++?
A base class in C++ is a class that serves as the foundation for derived classes. It contains common properties and behaviors that can be inherited by one or more derived classes. The base class provides the blueprint for creating objects, and the derived classes extend or specialize the base class by adding their unique properties and behaviors.
5. How do you define a base class in C++?
A base class in C++ is defined using the `class` keyword followed by the name of the base class. It can contain member variables, member functions, constructors, and destructors. Here’s an example:
```cpp class BaseClassName { // Member variables and member functions of the base class }; ```
6. What is single inheritance in C++?
Single inheritance in C++ is a type of inheritance where a derived class inherits from only one base class. It forms a simple hierarchical relationship between two classes, where the derived class inherits the properties of the single base class.
7. How do you implement single inheritance in C++?
Single inheritance in C++ is implemented by specifying the base class name after the colon (`:`) in the derived class declaration. Here’s an example:
```cpp class DerivedClassName : public BaseClassName { // Member variables and member functions of the derived class }; ```
8. What is multiple inheritance in C++?
Multiple inheritance in C++ is a type of inheritance where a derived class can inherit from multiple base classes. It allows a class to inherit properties and behaviors from more than one class simultaneously. With multiple inheritance, the derived class combines features from multiple base classes.
9. How do you implement multiple inheritance in C++?
Multiple inheritance in C++ is implemented by specifying multiple base class names separated by commas (`,`) after the colon (`:`) in the derived class declaration. Here’s an example:
```cpp class DerivedClassName : public BaseClass1, public BaseClass2 { // Member variables and member functions of the derived class }; ```
10. What is multilevel inheritance in C++?
Multilevel inheritance in C++ is a type of inheritance where a derived class is derived from another derived class. It forms a hierarchical chain of classes, where each derived class inherits properties from its immediate base class, which in
turn can be derived from another base class.
11. How do you implement multilevel inheritance in C++?
Multilevel inheritance in C++ is implemented by specifying the base class name after the colon (`:`) in the derived class declaration. This process can be repeated to create a chain of derived classes. Here’s an example:
```cpp class BaseClass { // Member variables and member functions of the base class }; class DerivedClass1 : public BaseClass { // Member variables and member functions of the derived class }; class DerivedClass2 : public DerivedClass1 { // Member variables and member functions of the derived class }; ```
12. What is hierarchical inheritance in C++?
Hierarchical inheritance in C++ is a type of inheritance where multiple derived classes are derived from a single base class. It forms a hierarchical structure with a common base class and multiple classes derived from it.
13. How do you implement hierarchical inheritance in C++?
Hierarchical inheritance in C++ is implemented by specifying the base class name after the colon (`:`) in each derived class declaration. Here’s an example:
```cpp class BaseClass { // Member variables and member functions of the base class }; class DerivedClass1 : public BaseClass { // Member variables and member functions of the derived class }; class DerivedClass2 : public BaseClass { // Member variables and member functions of the derived class }; ```
14. What is hybrid inheritance in C++?
Hybrid inheritance in C++ is a combination of multiple types of inheritance, such as single, multiple, multilevel, or hierarchical inheritance. It allows creating complex class hierarchies by combining different inheritance types.
15. How do you implement hybrid inheritance in C++?
Hybrid inheritance in C++ is implemented by combining different types of inheritance in a class hierarchy. It can involve using multiple base classes with different inheritance types, such as single, multiple, multilevel, or hierarchical inheritance.
16. What is the difference between public, private, and protected inheritance in C++?
In C++, the access specifiers (`public`, `private`, and `protected`) determine the visibility and accessibility of the inherited base class members in the derived class. The differences between these access specifiers are as follows:
– Public Inheritance: In public inheritance, the public members of the base class become public members of the derived class, and the protected members of the base class become protected members of the derived class. The private members of the base class are not accessible directly by the derived class.
– Private Inheritance: In private inheritance, all members of the base class become private members of the derived class. This means that both the public and protected members of the base class become private members in the derived class. The private members of the base class are not accessible directly by the derived class.
– Protected Inheritance: In protected inheritance, the public and protected members of the base class become protected members of the derived class. This means that the public members of the base class are accessible as protected members in the derived class. The private members of the base class are not accessible directly by the derived class.
17. How do you define the access specifiers in a derived class in C++?
The access specifiers (`public`, `private`, and `protected`) in a derived class can be defined before the base class name in the derived class declaration. By default, if no access specifier is specified, the access level is `private`. Here’s an example:
```cpp class DerivedClassName : access_specifier BaseClassName { // Member variables and member functions of the derived class }; ```
Replace `access_specifier` with `public`, `private`, or `protected` based on the desired access level.
18. How do you access base class members in a derived class in C++?
Base class members can be accessed in a derived class using the dot (`.`) operator or the scope resolution operator (`::`) followed by the base class name. The specific access depends on the access specifiers used. Here’s an example:
```cpp class BaseClass { public: int publicMember; private: int privateMember; protected: int protectedMember; }; class DerivedClass : public BaseClass { public: void accessBaseMembers() { publicMember = 1; // Access public member directly privateMember = 2; // Not accessible protectedMember = 3; // Access protected member directly } }; ```
19. What is the difference between a derived class and a base class in C++?
In C++, a derived class is a class that inherits properties and behaviors from another class called the base class. The derived class extends or specializes the base class by adding its unique properties and behaviors. The key differences between a derived class and a base class are:
– A derived class inherits the members (variables and functions) of the base class, while the base class does not inherit from any other class.
– The derived class can add additional members or modify the behavior of the inherited members, whereas the base class remains unchanged.
– Objects of the derived class can be treated as objects of the base class, but objects of the base class cannot be treated as objects of the derived class.
– The derived class forms a more specialized version of the base class and may have additional functionality or behavior.
20. How do you create an object of a derived class in C++?
To create an object of a derived class in C++, you can simply use the class name and call the constructor of the derived class. The constructor of the derived class implicitly calls the constructor of the base class. Here’s an example:
```cpp class BaseClass { // Base class definition }; class DerivedClass : public BaseClass { // Derived class definition }; int main() { DerivedClass obj; // Creating an object of the derived class // ... return 0; } ```
21. What is a constructor in a derived class in C++?
A constructor in a derived class is a special member function that is called when an object of the derived class is created. It is used to initialize the derived class object and its base class subobject. The derived class constructor can invoke the base class constructor to initialize the base class members.
22. How do you define and implement a constructor in a derived class in C++?
A constructor in a derived class is defined and implemented similar to a regular member function, but with the same name as the derived class. It can invoke the base class constructor using the constructor initializer list. Here’s an example:
```cpp class BaseClass { public: BaseClass(int value) { // Base class constructor code } }; class DerivedClass : public BaseClass { public: DerivedClass(int value) : BaseClass(value) { // Derived class constructor code } }; ```
In the example, the constructor of the derived class `DerivedClass` invokes the constructor of the base class `BaseClass` using the constructor initializer list `: BaseClass(value)`.
23. What is a destructor in a derived class in C++?
A destructor in a derived class is a special member function that is called when an object of the derived class is destroyed. It is used to perform cleanup tasks and deallocate resources used by the derived class and its base class.
24. How do you define and implement a destructor in a derived class in C++?
A destructor in a derived class is defined and implemented similar to a regular member function, but with the same name as the derived class preceded by a tilde (`~`). It can invoke the base class destructor implicitly to perform cleanup tasks. Here’s an example:
```cpp class BaseClass { public: ~BaseClass() { // Base class destructor code } }; class DerivedClass : public BaseClass { public: ~DerivedClass() { // Derived class destructor code } }; ```
In the example, the destructor of the derived class `DerivedClass` automatically calls the destructor of the base class `BaseClass` as part of the cleanup process.
25. Can a derived class access private members of a base class in C++?
No, a derived class cannot access the private members of a base class directly. Private members are only accessible within the scope of the base class itself. However, the derived class can access the inherited private members indirectly through public or protected member functions of the base class.
26. Can a derived class override a base class member function in C++?
Yes, a derived class can override a base class member function in C++. Function overriding is the ability of a derived class to provide a different implementation for a virtual member function declared in the base class. The function signature (return type, name, and parameters) must match in the base and derived classes for successful overriding.
27. How do you override a base class member function in a derived class in C++?
To override a base class member function in a derived class, you need to declare the function with the same signature (return type, name, and parameters) as the base class member function. Additionally, you need to use the `override` keyword to indicate that the function is intended to override a virtual function from the base class. Here’s an example:
```cpp class BaseClass { public: virtual void memberFunction() { // Base class member function implementation } }; class DerivedClass : public BaseClass { public: void memberFunction() override { // Derived class member function implementation } }; ```
In the example, the `DerivedClass` overrides the `memberFunction` of the `BaseClass` by declaring a function with the same signature and using the `override` keyword.
28. Can a derived class add new member functions that are not present in the base class in C++?
Yes, a derived class can add new member functions that are not present in the base class. Derived classes can extend the functionality of the base class by adding new member functions specific to the derived class. These new member functions can be accessed only through objects of the derived class.
29. Can a derived class add new member variables that are not present in the base class in C++?
Yes, a derived class can add new member variables that are not present in the base class. Derived classes can have their unique member variables in addition to the inherited member variables from the base class. These new member variables can be accessed only through objects of the derived class.
30. Can a derived class have the same member variables as the base class in C++?
Yes, a derived class can have the same member variables as the base class. The derived class inherits the member variables from the base class, and it can also define its own member
variables. If the derived class defines a member variable with the same name as the base class, it hides the base class member variable. However, the base class member variable can still be accessed using the scope resolution operator (`::`) with the base class name.
31. What is function hiding in C++?
Answer: Function hiding in C++ occurs when a derived class defines a function with the same name as a function in the base class, but the functions have different signatures. The derived class function “hides” the base class function, and the base class function becomes inaccessible through objects of the derived class.
32. How do you hide a base class function in a derived class in C++?
Answer: To hide a base class function in a derived class, you can define a function in the derived class with the same name but a different signature than the base class function. This will cause the base class function to be hidden in the derived class. You can use the `using` keyword to bring the hidden base class function into the derived class scope if needed.
33. What is the difference between function hiding and function overriding in C++?
Answer: In C++, function hiding and function overriding are two different concepts:
– Function hiding occurs when a derived class defines a function with the same name as a function in the base class but with a different signature. The base class function is hidden and not accessible through objects of the derived class. Function hiding is static and determined at compile-time.
– Function overriding occurs when a derived class provides a different implementation for a virtual function declared in the base class. The function signature must be the same in both the base and derived classes. Function overriding is dynamic and determined at runtime based on the actual type of the object.
34. What is a virtual base class in C++?
Answer: A virtual base class in C++ is a base class that is declared as virtual in a class hierarchy. When a class is derived from a virtual base class, the virtual base class subobject is shared among all the derived classes. This ensures that there is only one instance of the virtual base class within the complete object hierarchy.
35. How do you declare a virtual base class in C++?
Answer: To declare a virtual base class in C++, you add the `virtual` keyword before the base class name in the derived class declaration. For example:
```cpp class DerivedClass : virtual public BaseClass { // Derived class definition }; ```
In this example, the `virtual` keyword before the base class `BaseClass` indicates that it is a virtual base class.
36. What is the purpose of a virtual base class in C++?
Answer: The purpose of a virtual base class in C++ is to avoid the problems associated with multiple inheritance, such as the “diamond problem.” By declaring a base class as virtual, it ensures that only a single instance of that base class exists in the complete object hierarchy, even if it is inherited by multiple paths. This prevents duplicate copies of the virtual base class and ensures proper initialization and sharing of common data.
37. What is the diamond problem in multiple inheritance in C++?
Answer: The diamond problem is a name collision issue that arises in multiple inheritance when a class inherits from two or more classes that share a common base class. It leads to ambiguity in accessing the members of the common base class, as there are multiple paths to reach them. This can result in compilation errors or unexpected behavior.
38. How do you resolve the diamond problem in multiple inheritance in C++?
Answer: The diamond problem in multiple inheritance can be resolved in C++ by using virtual inheritance. By declaring the common base class as a virtual base class in the derived classes, it ensures that only a single instance of the base class exists in the complete object hierarchy. This resolves the ambiguity and allows proper access to the common base class members.
39. What is an abstract base class in C++?
Answer: An abstract base class in C++ is a class that is designed to be used as a base
class and cannot be instantiated on its own. It is often used to define a common interface or behavior that derived classes must implement. An abstract base class typically contains one or more pure virtual functions, making it an abstract class.
40. How do you define and use an abstract base class in C++?
Answer: To define an abstract base class in C++, you declare at least one pure virtual function in the class. A pure virtual function is declared using the `virtual` keyword and assigning it a value of `0` (zero) as its implementation. For example:
```cpp class AbstractBase { public: virtual void pureVirtualFunction() = 0; // Pure virtual function }; ```
To use an abstract base class, you derive a concrete class from it and provide implementations for all the pure virtual functions. The derived class becomes a concrete class and can be instantiated.
41. Can you create objects of an abstract base class in C++?
Answer: No, you cannot create objects of an abstract base class in C++. An abstract base class is designed to be used as a base class and cannot be instantiated on its own because it contains pure virtual functions that have no implementation. However, you can create objects of derived classes that inherit from the abstract base class.
42. What is a pure virtual function in C++?
Answer: A pure virtual function in C++ is a virtual function that is declared in a base class but has no implementation. It is declared using the `virtual` keyword and assigning it a value of `0` (zero) as its implementation. A pure virtual function serves as a placeholder that derived classes must override and provide an implementation for.
43. How do you declare and define a pure virtual function in C++?
Answer: To declare a pure virtual function in C++, you include the `virtual` keyword and assign it a value of `0` (zero) as its implementation. For example:
```cpp class BaseClass { public: virtual void pureVirtualFunction() = 0; // Pure virtual function }; ```
The pure virtual function has no implementation in the base class itself. It must be overridden and defined in the derived classes.
44. Can a pure virtual function have a definition in C++?
Answer: No, a pure virtual function cannot have a definition in C++. It is meant to be overridden and implemented in derived classes. However, it is possible to provide a default implementation for a pure virtual function by defining a non-pure virtual function with the same name in the base class. Derived classes can choose to override this non-pure virtual function or use the default implementation.
45. What is the difference between an abstract base class and a concrete base class in C++?
Answer: In C++, an abstract base class is a class that is designed to be used as a base class and cannot be instantiated on its own. It typically contains one or more pure virtual functions. A concrete base class, on the other hand, is a regular class that can be instantiated and used on its own, without necessarily having any pure virtual functions.
46. What is a constructor initializer list in a derived class in C++?
Answer: A constructor initializer list in a derived class in C++ is used to invoke the base class constructor and initialize the base class subobject within the derived class constructor. It specifies the arguments to be passed to the base class constructor and any additional initialization for member variables in the derived class.
47. How do you use a constructor initializer list in a derived class in C++?
Answer: To use a constructor initializer list in a derived class in C++, you include the base class name followed by parentheses and pass the necessary arguments to the base class constructor. For example:
```cpp DerivedClass::DerivedClass(int arg1 , int arg2) : BaseClass(arg1), memberVariable(arg2) { // Additional initialization code for derived class } ```
In this example, the constructor initializer list `: BaseClass(arg1)` initializes the base class using the `arg1` argument, and `memberVariable(arg2)` initializes the member variable `memberVariable` with the `arg2` argument.
48. What is the purpose of a constructor initializer list in a derived class in C++?
Answer: The purpose of a constructor initializer list in a derived class in C++ is to ensure that the base class constructor is called with the appropriate arguments and that the base class subobject is properly initialized before the derived class constructor body is executed. It allows you to initialize the base class and member variables in a concise and efficient manner.
49. What is a protected access specifier in C++?
Answer: In C++, the protected access specifier defines the access level for class members that are accessible within the class itself and its derived classes. Protected members are not accessible outside the class hierarchy but can be accessed by derived classes.
50. How do you use the protected access specifier in a derived class in C++?
Answer: To use the protected access specifier in a derived class in C++, you declare the desired member variables or functions with the `protected` keyword within the base class. These protected members can then be accessed directly by the derived class, just like private members, but are also accessible by other derived classes.
51. What is the difference between public and protected inheritance in C++?
Answer: In C++, public inheritance and protected inheritance are different ways of specifying the access level for inherited members:
– Public inheritance (`public` keyword) preserves the access levels of base class members in the derived class. Public members of the base class remain public in the derived class, protected members become protected, and private members are inaccessible.
– Protected inheritance (`protected` keyword) changes the access level of all members inherited from the base class to protected in the derived class. Public and protected members of the base class become protected in the derived class, while private members remain inaccessible.
52. What is the difference between protected and private inheritance in C++?
Answer: In C++, protected inheritance and private inheritance are similar in that they both change the access level of inherited members:
– Protected inheritance (`protected` keyword) changes the access level of all members inherited from the base class to protected in the derived class. Public and protected members of the base class become protected in the derived class, while private members remain inaccessible.
– Private inheritance (`private` keyword) changes the access level of all members inherited from the base class to private in the derived class. Both public and protected members of the base class become private in the derived class, and private members are inaccessible.
53. What is the use of the ‘using’ keyword in C++ inheritance?
Answer: The ‘using’ keyword in C++ inheritance is used to bring base class member functions or variables into the scope of the derived class. It allows the derived class to access and use the base class members as if they were its own.
54. How do you use the ‘using’ keyword to bring base class member functions into the derived class in C++?
Answer: To use the ‘using’ keyword to bring base class member functions into the derived class in C++, you include a ‘using’ declaration in the derived class for the desired base class member function. For example:
```cpp class Derived : public Base { public: using Base::baseFunction; // Bring baseFunction into derived class scope }; ```
In this example, the ‘using’ declaration ‘using Base::baseFunction;’ brings the ‘baseFunction’ from the base class ‘Base’ into the scope of the derived class
‘Derived’.
55. What is the scope resolution operator (::) used for in C++ inheritance?
Answer: The scope resolution operator (::) in C++ inheritance is used to explicitly specify the scope from which a particular member function or variable is accessed. It can be used to access members of the base class from within the derived class, or to access members of the derived class from outside its scope.
56. How do you access base class members using the scope resolution operator in C++ inheritance?
Answer: To access base class members using the scope resolution operator in C++ inheritance, you specify the name of the base class followed by the scope resolution operator (::) and the name of the member. For example:
```cpp void Derived::someFunction() { Base::baseFunction(); // Access base class member function int value = Base::baseVariable; // Access base class member variable } ```
In this example, ‘Base::baseFunction()’ and ‘Base::baseVariable’ access the base class member function and member variable, respectively, from within the derived class ‘Derived’.
57. How do you access derived class members using the scope resolution operator in C++ inheritance?
Answer: Since derived class members are accessible within the derived class itself, there is no need to use the scope resolution operator (::) to access them from within the derived class. However, you can use the scope resolution operator to access derived class members from outside the derived class. For example:
```cpp Derived derivedObject; int value = derivedObject::derivedVariable; // Access derived class member variable ```
In this example, ‘derivedObject::derivedVariable’ accesses the member variable ‘derivedVariable’ of the derived class ‘Derived’ from outside its scope.
58. What is a friend class in C++ inheritance?
Answer: A friend class in C++ inheritance is a class that is granted access to the private and protected members of another class. A friend class can access the private and protected members of the class it is declared as a friend of, even though it is not derived from that class.
59. How do you declare a friend class in C++ inheritance?
Answer: To declare a friend class in C++ inheritance, you include a ‘friend’ declaration inside the class that you want to grant access to. For example:
```cpp class FriendClass { // FriendClass definition }; class MyClass { friend class FriendClass; // Declare FriendClass as a friend class // MyClass definition }; ```
In this example, ‘FriendClass’ is declared as a friend class of ‘MyClass’, granting it access to the private and protected members of ‘MyClass’.
60. What is the difference between a friend class and a base class in C++ inheritance?
Answer: In C++ inheritance, a friend class and a base class are different concepts:
– A friend class is a class that is granted access to the private and protected members of another class. It is not necessarily related through inheritance but is explicitly declared as a friend of the class.
– A base class, on the other hand, is a class from which another class (derived class) is derived. The derived class inherits the members and properties of the base class, including public, protected, and accessible private members.
61. What is a derived class constructor in C++ inheritance?
Answer: A derived class constructor is a special member function in a derived class that is responsible for initializing the derived class objects. It is called when an object of the derived class is created and is used to initialize the data members inherited from the base class as well as the additional data members specific to the derived class.
62. How do you define and implement a derived class constructor in C++ inheritance?
Answer: To define and implement a derived class constructor in C++ inheritance, you can use the constructor initializer list to initialize the base class subobject and specify any additional initialization steps for the derived class. Here’s an example:
```cpp class Base { public: Base(int value) { // Base class constructor implementation } }; class Derived : public Base { public: Derived(int value1, int value2) : Base(value1) { // Derived class constructor implementation // Additional initialization steps for Derived } }; ```
63. What is the order of execution of base class and derived class constructors in C++ inheritance?
Answer: In C++ inheritance, the base class constructor is executed before the derived class constructor. This ensures that the base class subobject is properly initialized before any initialization specific to the derived class takes place. The order of execution is from the topmost base class to the most derived class.
64. What is a derived class destructor in C++ inheritance?
Answer: A derived class destructor is a special member function in a derived class that is responsible for cleaning up the resources allocated by the derived class objects. It is called when an object of the derived class goes out of scope or is explicitly deleted and is used to release the resources held by the derived class and its base class.
65. How do you define and implement a derived class destructor in C++ inheritance?
Answer: To define and implement a derived class destructor in C++ inheritance, you can simply provide the destructor definition within the derived class. The destructor will automatically call the base class destructor to ensure proper cleanup of resources. Here’s an example:
```cpp class Base { public: ~Base() { // Base class destructor implementation } }; class Derived : public Base { public: ~Derived() { // Derived class destructor implementation // Additional cleanup steps for Derived } }; ```
66. What is the order of execution of derived class and base class destructors in C++ inheritance?
Answer: In C++ inheritance, the derived class destructor is executed before the base class destructor. This ensures that the cleanup operations specific to the derived class are performed before the base class cleanup. The order of execution is from the most derived class to the topmost base class.
67. What is the difference between a derived class constructor and a derived class destructor in C++ inheritance?
Answer: The main difference between a derived class constructor and a derived class destructor in C++ inheritance is their purpose and functionality.
– Constructor: A derived class constructor is responsible for initializing the derived class objects, including initializing the base class subobject and any additional data members specific to the derived class. It is called when an object of the derived class is created.
– Destructor: A derived class destructor is responsible for cleaning up the resources allocated by the derived class objects, including releasing the resources held by the derived class and its base class. It is called when an object of the derived class goes out of scope or is explicitly deleted.
68. What is a protected constructor in C++ inheritance?
Answer: A protected constructor in C++ inheritance is a constructor that is accessible within the derived class and its derived classes but not accessible outside the class hierarchy. It allows derived classes to invoke the constructor for initialization purposes, but prevents direct construction of
objects of the base or derived classes from outside the class hierarchy.
69. How do you declare a protected constructor in C++ inheritance?
Answer: To declare a protected constructor in C++ inheritance, you can use the `protected` access specifier before the constructor declaration within the class. Here’s an example:
```cpp class Base { protected: Base() { // Protected constructor } }; class Derived : public Base { public: Derived() : Base() { // Derived class constructor invoking the protected base class constructor } }; ```
70. What is the use of a protected constructor in C++ inheritance?
Answer: The use of a protected constructor in C++ inheritance is to enforce encapsulation and control the access to object creation within the class hierarchy. By declaring a constructor as protected, it allows derived classes to access and invoke the constructor for initialization, but restricts direct construction of objects of the base or derived classes from outside the class hierarchy.
71. What is a static member in a derived class in C++ inheritance?
Answer: A static member in a derived class in C++ inheritance is a member that belongs to the class itself rather than to the individual objects of the class. It is shared among all the objects of the derived class and its derived classes, as well as the base class. Static members are declared using the `static` keyword and can be accessed without creating an object of the class.
72. How do you declare and define a static member in a derived class in C++ inheritance?
Answer: To declare and define a static member in a derived class in C++ inheritance, you can use the `static` keyword in the class declaration and provide the definition outside the class. Here’s an example:
```cpp class Base { public: static int count; // Declaration of static member }; int Base::count = 0; // Definition of static member class Derived : public Base { // Derived class definition }; ```
73. How do you access a static member of a base class in a derived class in C++ inheritance?
Answer: A static member of a base class can be accessed in a derived class using the scope resolution operator `::` along with the base class name. Here’s an example:
```cpp class Base { public: static int count; }; int Base::count = 0; class Derived : public Base { public: void printCount() { cout << "Count: " << Base::count << endl; // Accessing the static member of the base class } }; ```
74. How do you access a static member of a derived class in C++ inheritance?
Answer: A static member of a derived class can be accessed using the class name itself, without the need for an object. Here’s an example:
```cpp class Base { public: static int count; }; int Base::count = 0; class Derived : public Base { public: static void printCount() { cout << "Count: " << count << endl; // Accessing the static member of the derived class } }; ```
75. What is a virtual destructor in C++ inheritance?
Answer: A virtual destructor in C++ inheritance is a destructor declared in the base class as virtual. It allows proper destruction of derived class objects through a base class pointer or reference. When a derived class object is deleted through a base class pointer or reference, the appropriate derived class destructor is invoked along with the base class destructor.
76. How do you declare and define a virtual destructor in C++ inheritance?
Answer: To declare and define a virtual destructor in C++ inheritance, you can add the `virtual` keyword before the destructor declaration in the base class. Here’s an example:
“`
cpp class Base { public: virtual ~Base() { // Virtual destructor implementation } }; class Derived : public Base { public: ~Derived() { // Derived class destructor implementation } }; ```
77. What is the purpose of a virtual destructor in C++ inheritance?
Answer: The purpose of a virtual destructor in C++ inheritance is to ensure proper destruction of derived class objects when they are deleted through a base class pointer or reference. Without a virtual destructor, only the base class destructor would be called, resulting in potential memory leaks or undefined behavior. A virtual destructor allows the appropriate derived class destructor to be invoked along with the base class destructor during object destruction.
78. What is an object pointer in C++ inheritance?
Answer: An object pointer in C++ inheritance is a pointer that can hold the memory address of an object of a base class or a derived class. It allows accessing the members and invoking the member functions of the object through the pointer.
79. How do you declare and initialize an object pointer in C++ inheritance?
Answer: To declare and initialize an object pointer in C++ inheritance, you can declare a pointer of the appropriate class type and assign it the address of the object using the address-of operator `&`. Here’s an example:
```cpp Base* ptr = new Derived(); // Declaring and initializing an object pointer with a derived class object ```
80. How do you access base class members using an object pointer in C++ inheritance?
Answer: Base class members can be accessed using an object pointer in C++ inheritance by using the arrow operator `->` along with the pointer name. Here’s an example:
```cpp Base* ptr = new Derived(); // Object pointer to a derived class object ptr->baseMember(); // Accessing a base class member function ```
81. How do you access derived class members using an object pointer in C++ inheritance?
Answer: Derived class members can be accessed using an object pointer in C++ inheritance by first casting the pointer to the appropriate derived class type using the `static_cast` or `dynamic_cast` and then using the arrow operator `->` along with the pointer name. Here’s an example:
```cpp Base* ptr = new Derived(); // Object pointer to a derived class object Derived* derivedPtr = static_cast<Derived*>(ptr); // Casting to derived class type derivedPtr->derivedMember(); // Accessing a derived class member function ```
82. What is a dynamic cast in C++ inheritance?
Answer: A dynamic cast in C++ inheritance is a type of cast used to safely convert pointers or references of a base class to pointers or references of a derived class at runtime. It performs a runtime check to ensure the validity of the cast and returns a null pointer if the cast fails.
83. How do you perform a dynamic cast in C++ inheritance?
Answer: To perform a dynamic cast in C++ inheritance, you can use the `dynamic_cast` operator followed
by the target type enclosed in parentheses. Here’s an example:
```cpp Base* basePtr = new Derived(); // Object pointer to a derived class object Derived* derivedPtr = dynamic_cast<Derived*>(basePtr); // Dynamic cast from base class to derived class if (derivedPtr != nullptr) { // Cast is successful, access derived class members derivedPtr->derivedMember(); } ```
84. What is the purpose of a dynamic cast in C++ inheritance?
Answer: The purpose of a dynamic cast in C++ inheritance is to safely convert pointers or references of a base class to pointers or references of a derived class at runtime. It allows runtime type checking to ensure the validity of the cast and provides a way to access derived class members when working
with polymorphic objects.
85. What is a static cast in C++ inheritance?
Answer: A static cast in C++ inheritance is a type of cast used to perform simple conversions between related types, such as casting a pointer or reference of a base class to a pointer or reference of a derived class. It is resolved at compile-time and does not perform any runtime checks.
86. How do you perform a static cast in C++ inheritance?
Answer: To perform a static cast in C++ inheritance, you can use the `static_cast` operator followed by the target type enclosed in parentheses. Here’s an example:
```cpp Base* basePtr = new Derived(); // Object pointer to a derived class object Derived* derivedPtr = static_cast<Derived*>(basePtr); // Static cast from base class to derived class ```
87. What is the purpose of a static cast in C++ inheritance?
Answer: The purpose of a static cast in C++ inheritance is to perform simple conversions between related types, such as casting a pointer or reference of a base class to a pointer or reference of a derived class. It is useful when you know the types involved and want to perform explicit type conversion at compile-time.
88. What is an upcast in C++ inheritance?
Answer: An upcast in C++ inheritance is a type of cast that converts a pointer or reference of a derived class to a pointer or reference of its base class. It is a safe and implicit conversion, as the derived class is guaranteed to have all the members and functionality of the base class.
89. How do you perform an upcast in C++ inheritance?
Answer: An upcast in C++ inheritance is automatically performed when assigning a pointer or reference of a derived class to a pointer or reference of its base class. No explicit cast is required. Here’s an example:
```cpp Derived derivedObj; Base* basePtr = &derivedObj; // Upcast from derived class to base class ```
90. What is the purpose of an upcast in C++ inheritance?
Answer: The purpose of an upcast in C++ inheritance is to treat a derived class object as an object of its base class. It allows accessing the base class members and functionality of the derived class object through a base class pointer or reference.
91. What is a downcast in C++ inheritance?
Answer: A downcast in C++ inheritance is a type of cast that converts a pointer or reference of a base class to a pointer or reference of a derived class. It is a potentially unsafe conversion, as the base class may not have the same members and functionality as the derived class.
92. How do you perform a downcast in C++ inheritance?
Answer: To perform a downcast in C++ inheritance, you can use either a `static_cast` or a `dynamic_cast` operator. However, it is generally recommended to use `dynamic_cast` for downcasting, as it performs a runtime check to ensure the validity of the cast. Here’s an example:
```cpp Base* basePtr = new Derived(); // Object pointer to a base class object Derived* derivedPtr = dynamic_cast<Derived*>(basePtr); // Downcast from base class to derived class if (derivedPtr != nullptr) { // Cast is successful, access derived class members derivedPtr->derivedMember(); } ```
93. What is the purpose of a downcast in C++ inheritance?
Answer: The purpose of a downcast in C++ inheritance is to explicitly convert a pointer or reference of a base class to a pointer or reference of a derived class. It allows accessing the members and functionality specific to the derived class when necessary. However, downcasting should be used with caution and preferably with runtime type checking to ensure
the validity of the cast.
94. What is a pure virtual destructor in C++ inheritance?
Answer: A pure virtual destructor in C++ inheritance is a destructor declared as pure virtual in the base class. It is declared using the `virtual` keyword and assigning it the value `0` in the base class. A class with a pure virtual destructor is considered an abstract class and cannot be instantiated.
95. How do you declare and define a pure virtual destructor in C++ inheritance?
Answer: To declare and define a pure virtual destructor in C++ inheritance, you can add the `virtual` keyword before the destructor declaration in the base class and assign it the value `0`. Here’s an example:
```cpp class Base { public: virtual ~Base() = 0; // Declaration of pure virtual destructor }; Base::~Base() { // Definition of pure virtual destructor } class Derived : public Base { public: ~Derived() { // Derived class destructor implementation } }; ```
96. Can a pure virtual destructor have a definition in C++ inheritance?
Answer: Yes, a pure virtual destructor can have a definition in C++ inheritance. The definition is provided outside the class similar to other member functions. The pure virtual destructor in the base class forces the derived classes to provide their own definitions for the destructor.
97. What is an abstract derived class in C++ inheritance?
Answer: An abstract derived class in C++ inheritance is a class that inherits from an abstract base class and has not provided definitions for all the pure virtual functions inherited from the base class. An abstract derived class is also considered an abstract class and cannot be instantiated.
98. How do you define and use an abstract derived class in C++ inheritance?
Answer: To define and use an abstract derived class in C++ inheritance, you can inherit from an abstract base class and provide definitions for all the pure virtual functions inherited from the base class. The derived class can then be used to create objects and invoke its member functions.
99. Can you create objects of an abstract derived class in C++ inheritance?
Answer: No, you cannot create objects of an abstract derived class in C++ inheritance. An abstract derived class is an incomplete class that has not provided definitions for all the pure virtual functions inherited from the base class. It is meant to be used as a base for further derived classes and cannot be instantiated.
100. What is the difference between an abstract derived class and a concrete derived class?
Answer: The difference between an abstract derived class and a concrete derived class lies in the level of completeness and the ability to instantiate objects.
– An abstract derived class is a class that inherits from an abstract base class and has not provided definitions for all the pure virtual functions inherited from the base class. It is an incomplete class and cannot be instantiated. It is used as a base for further derived classes that provide implementations for the pure virtual functions.
– A concrete derived class, on the other hand, is a class that inherits from a base class and provides definitions for all the inherited pure virtual functions. It is a complete class that can be instantiated and used to create objects. It adds specific functionality to the base class and can be further extended by additional derived classes.
In summary, an abstract derived class is incomplete and cannot be instantiated, while a concrete derived class is complete and can be instantiated.