What is mean by data structure ? Lets have brief introduction about data structure, Lets us takes an example ,in our room we have different things but scattered in different way, if we can place those things in proper way ,we can find the things in fastest way and in proper time…The Same way Data […]
Data structure Tutorials
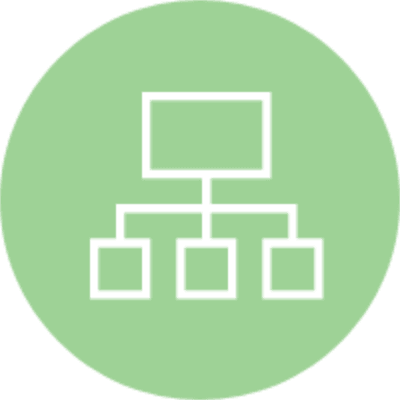
In computer science, a data structure is a way of organizing and storing data in a computer program so that it can be accessed and used efficiently. It defines the relationship between the data, the operations that can be performed on the data, and the algorithms that are used to implement those operations.
In programming, understanding data structures is crucial for efficient and effective programming. It allows developers to manipulate and process large amounts of data efficiently, which is important for many applications in fields such as data analysis, machine learning, and web development.
There are various types of data structures, such as arrays, linked lists, stacks, queues, trees, and graphs, each with its own advantages and disadvantages depending on the use case.
In learning about data structures, one will learn about the different types of data structures and their properties, how to implement and use them, and how to choose the appropriate data structure for a given problem.
The importance of data structures in programming lies in their ability to optimize the performance of programs by reducing the time and space complexity of algorithms. By choosing the right data structure for a specific problem, developers can ensure that their code runs efficiently and effectively, even for large datasets. Additionally, a good understanding of data structures can make it easier to write and maintain code, as well as debug it when issues arise.
By the end of the data structure learning journey, you will have a solid understanding of the various types of data structures and their properties, as well as the algorithms used to manipulate them.
Throughout your learning journey, you will also learn how to analyze the time and space complexity of algorithms that use these data structures, as well as how to choose the appropriate data structure for a given problem. Additionally, you will gain hands-on experience implementing data structures and algorithms in various programming languages, which will give you the skills needed to build efficient and effective software applications.
Specifically, you will learn about:
What is An Algorithm? An algorithm is a sequence of unambiguous instructions which can be used to solve the problem . In addition every algorithm must satisfy the following criteria: Input: there are zero or more quantities, which are externally supplied; Output: at least one quantity is produced; Definiteness: each instruction must be clear and […]
Overview of Recursion: A function must be able to call itself. For example, let us consider the function factr() shown below, which computers the factorial of an integer. #include < stdio.h > int factorial (int); main() { int num, fact; printf (“Enter a positive integer value: “); scanf (“%d”, &num); fact = factorial (num); printf […]
Differences between recursion and iteration: Both involve repetition. Both involve a termination test. Both can occur infinitely.
in The Previous Post We have Learned What is algorithm? In Computer Science we have some problem which we have to solve, but before writing the actual program, we can write in informal language ,that is called Algorithm, But Before implementing Algorithm in actual sense we have to take care of how much time and […]
In This section lets understand the Basic concept of NP Hard & NP Complete Algorithm NP-Hard and NP-Complete Problems An algorithm A is of polynomial complexity is there exist a polynomial p( ) such that the computing time of A is O(p(n)). Definition: P is a set of all decision problems solvable by a deterministic […]
In this Post, the list data structure is presented. This structure can be used as the basis for the implementation of other data structures (stacks, queues etc.). The basic linked list can be used without modification in many programs. However, some applications require enhancements to the linked list design. These enhancements fall into three broad […]
Linked List Concepts A linked list is a non-sequential collection of data items. It is a dynamic data structure. For every data item in a linked list, there is an associated pointer that would give the memory location of the next data item in the linked list. The data items in the linked list are […]
Single Linked List In Brief: A linked list allocates space for each element separately in its own block of memory called a “node”. The list gets an overall structure by using pointers to connect all its nodes together like the links in a chain. Each node contains two fields; a “data” field to store whatever […]
Another alternative is to allocate the nodes in blocks. In fact, if you know the maximum size of a list a head of time, you can pre-allocate the nodes in a single array. The result is a hybrid structure – an array based linked list. Figure 3.5.1 shows an example of null terminated single linked […]
A double linked list is a two-way list in which all nodes will have two links. This helps in accessing both successor node and predecessor node from the given node position. It provides bi-directional traversing. Each node contains three fields: Left link. Data. Right link. The left link points to the predecessor node and the […]
Concept: It is just a single linked list in which the link field of the last node points back to the address of the first node. A circular linked list has no beginning and no end. It is necessary to establish a special pointer called start pointer always pointing to the first node of the […]
Concept: A circular double linked list has both successor pointer and predecessor pointer in circular manner. The objective behind considering circular double linked list is to simplify the insertion and deletion operations performed on double linked list. In circular double linked list the right link of the right most node points back to the start […]
The major disadvantage of doubly linked lists (over singly linked lists) is that they require more space (every node has two pointer fields instead of one). Also, the code to manipulate doubly linked lists needs to maintain the prev fields as well as the next fields; the more fields that have to be maintained, the […]
Data structure Stack:There are certain situations in computer science that one wants to restrict insertions and deletions so that they can take place only at the beginning or the end of the list, not in the middle. Two of such data structures that are useful are: Stack. Queue. Linear lists and arrays allow one to […]
An algebraic expression is a legal combination of operators and operands. Operand is the quantity on which a mathematical operation is performed. Operand may be a variable like x, y, z or a constant like 5, 4, 6 etc. Operator is a symbol which signifies a mathematical or logical operation between the operands. Examples of […]
A queue is another special kind of list, where items are inserted at one end called the rear and deleted at the other end called the front. Another name for a queue is a “FIFO” or “First-in-first-out” list. The operations for a queue are analogues to those for a stack, the difference is that the […]
A more efficient queue representation is obtained by regarding the array Q[MAX] as circular. Any number of items could be placed on the queue. This implementation of a queue is called a circular queue because it uses its storage array as if it were a circle instead of a linear list. There are two problems […]
In the preceding section we saw that a queue in which we insert items at one end and from which we remove items at the other end. In this section we examine an extension of the queue,Deque ,which provides a means to insert and remove items at both ends of the queue. This data structure […]
A priority queue is a collection of elements such that each element has been assigned a priority and such that the order in which elements are deleted and processed comes from the following rules: An element of higher priority is processed before any element of lower priority. two elements with same priority are processed according […]
A data structure is said to be linear if its elements form a sequence or a linear list. Previous linear data structures that we have studied like an array, stacks, queues and linked lists organize data in linear order. A data structure is said to be non linear if its elements form a hierarchical classification […]
Binary Tree Traversal Techniques: A tree traversal is a method of visiting every node in the tree. By visit, we mean that some type of operation is performed. For example, you may wish to print the contents of the nodes. There are four common ways to traverse a binary tree:d Preorder Inorder Postorder Level order […]
The linked representation of any binary tree has more null links than actual pointers. If there are 2n total links, there are n+1 null links. A clever way to make use of these null links has been devised by A.J. Perlis and C. Thornton. Their idea is to replace the null links by pointers called […]
A binary search tree is a binary tree. It may be empty. If it is not empty then it satisfies the following properties: Every element has a key and no two elements have the same key. The keys in the left subtree are smaller than the key in the root. The keys in the right […]
If in a tree, the outdegree of every node is less than or equal to m, the tree is called general tree. The general tree is also called as an m-ary tree. If the outdegree of every node is exactly equal to m or zero then the tree is called a full or complete m-ary […]
There is a one-to-one mapping between general ordered trees and binary trees. So, every tree can be uniquely represented by a binary tree. Furthermore, a forest can also be represented by a binary tree. Conversion from general tree to binary can be done in two stages. Stage 1: As a first step, we delete all […]
Search involves visiting nodes in a tree in a systematic manner, and may or may not result into a visit to all nodes. When the search necessarily involved the examination of every vertex in the tree, it is called the traversal. Traversing of a tree can be done in two ways. Depth first search or […]
Graph G is a pair (V, E), where V is a finite set of vertices and E is a finite set of edges. We will often denote n = |V|, e = |E|. A graph is generally displayed as figure 6.5.1, in which the vertices are represented by circles and the edges by lines. An […]
A spanning tree for a connected graph is a tree whose vertex set is the same as the vertex set of the given graph, and whose edge set is a subset of the edge set of the given graph. i.e., any connected graph will have a spanning tree. Weight of a spanning tree w(T) is […]
Many graph algorithms require one to systematically examine the nodes and edges of a graph G. There are two standard ways to do this. They are: Breadth first traversal (BFT) Depth first traversal (DFT) The BFT will use a queue as an auxiliary structure to hold nodes for future processing and the DFT will use […]
There are basically two aspects of computer programming. One is data organization also commonly called as data structures. Till now we have seen about data structures and the techniques and algorithms used to access them. The other part of computer programming involves choosing the appropriate algorithm to solve the problem. Data structures and algorithms are […]
This is the simplest of all searching techniques. In this technique, an ordered or unordered list will be searched one by one from the beginning until the desired element is found. If the desired element is found in the list then the search is successful otherwise unsuccessful. Suppose there are ‘n’ elements organized sequentially on […]
If we have ‘n’ records which have been ordered by keys so that x1 < x2 < … < xn . When we are given a element ‘x’, binary search is used to find the corresponding element from the list. In case ‘x’ is present, we have to determine a value ‘j’ such that a[j] […]
The bubble sort is easy to understand and program. The basic idea of bubble sort is to pass through the file sequentially several times. In each pass, we compare each element in the file with its successor i.e., X[i] with X[i+1] and interchange two element when they are not in proper order. We will illustrate […]
Selection sort will not require no more than n-1 interchanges. Suppose x is an array of size n stored in memory. The selection sort algorithm first selects the smallest element in the array x and place it at array position 0; then it selects the next smallest element in the array x and place it […]
The quick sort was invented by Prof. C. A. R. Hoare in the early 1960’s. It was one of the first most efficient sorting algorithms. It is an example of a class of algorithms that work by “divide and conquer” technique. The quick sort algorithm partitions the original array by rearranging it into two groups. […]
Heap is a data structure, which permits one to insert elements into a set and also to find the largest element efficiently. A data structure, which provides these two operations, is called a priority queue. Max and Min Heap data structures: A max heap is an almost complete binary tree such that the value of […]
Here on design and analysis of algorithms today’s it is going to be an overview of the course and i want to introduce you to the main problems in the course and also convey a spirit of the course let us start with the family question given a certain problem how do you solve it in a […]
Dijkstra’s algorithm finds the shortest paths from a given node to all other nodes in a graph Initially, Mark the given node as known (path length is zero)For each out-edge, set the distance in each neighboring node equal to the cost (length) of the out-edge, and set its predecessor to the initially given node Repeatedly […]
The Dynamic Programming (DP) is the most powerful design technique for solving optimization problems. It was invented by mathematician named Richard Bellman inn 1950s. The DP in closely related to divide and conquer techniques, where the problem is divided into smaller sub-problems and each sub-problem is solved recursively. The DP differs from divide and conquer in a way […]
DIVIDE AND CONQUER ALGORITHM In this approach ,we solve a problem recursively by applying 3 steps DIVIDE-break the problem into several sub problems of smaller size. CONQUER-solve the problem recursively COMBINE-combine these solutions to create a solution to the original problem.
This is a greedy algorithm. A greedy algorithm chooses some local optimum (i.e. picking an edge with the least weight in a MST). Kruskal’s algorithm works as follows: Take a graph with ‘n’ vertices, keep on adding the shortest (least cost) edge, while avoiding the creation of cycles, until (n – 1) edges have been […]
A given graph can have many spanning trees. From these many spanning trees, we have to select a cheapest one. This tree is called as minimal cost spanning tree. Minimal cost spanning tree is a connected undirected graph G in which each edge is labeled with a number (edge labels may signify lengths, weights other […]